Table of Contents
Introduction
In the previous article, we created a database using the Entity Framework Code First approach, which has proven to be a powerful tool for creating and managing databases.
In this article, we will implement the Generic Repository Pattern C# With Entity Framework Core for the database created in the previous post.
What are Design Patterns?
Design patterns describe the generic solutions to software design problems. Imagine you have been given the task of designing a solution for a software system. The probability that someone else has designed a similar solution in the past is high, which means that a generic solution or approach to the same problem already exists, and all you need is to apply this pattern to solve your software design problems.
What is the Generic Repository Pattern C#?
The repository pattern is a design pattern in software development that is used to abstract the data access logic by encapsulating the data access layer for communication with the data layer so that the consumer does not have to worry about the ORM used, making it easier to work with different types of data sources and making the code reusable, testable and maintainable, as shown in the following sequence diagram.
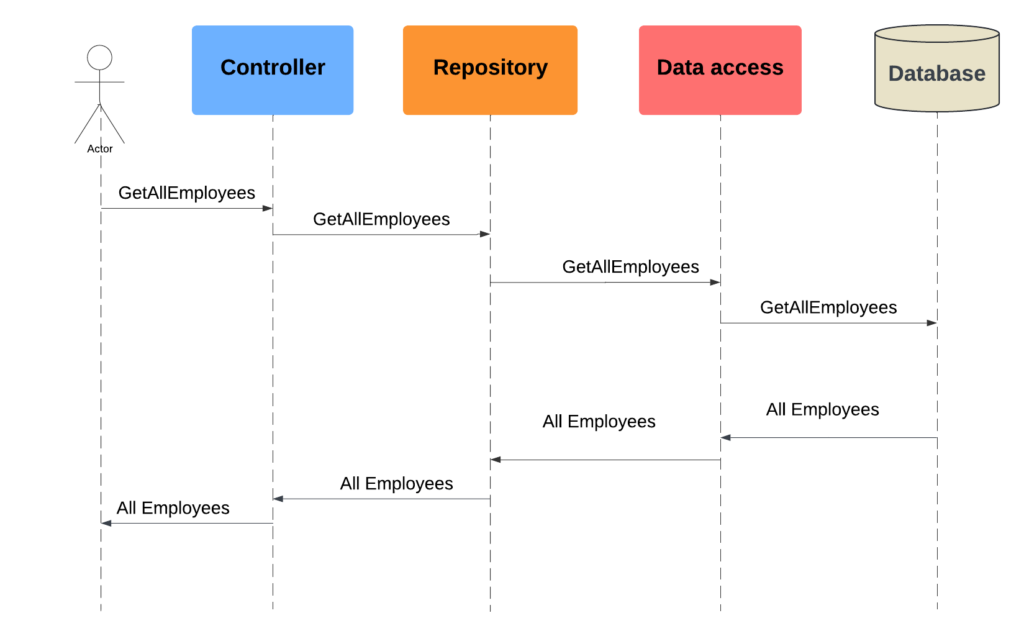
We implement the repository pattern by defining an interface contract that can handle CRUD operations for each entity type, and each repository of each entity type must implement its interface, resulting in repeatable code and redundancy, as shown in the following class diagram.
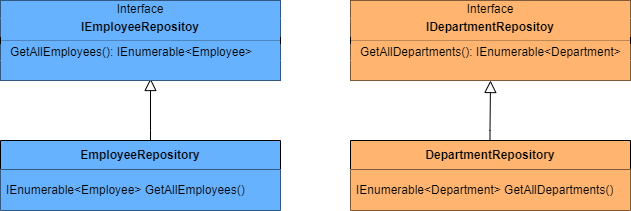
While the generic repository pattern simplifies the repository pattern by creating a single generic repository that can handle CRUD operations for each entity type, instead of having a separate repository for each entity, as shown in the following class diagram.
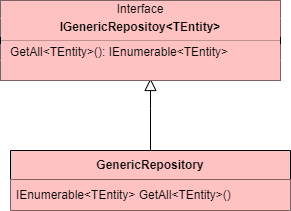
Pros and Cons of Generic Repository Pattern C#
If your software is simple and you don’t need to do anything special and the CRUD operations fit, then the generic repository pattern is a good choice for you and you can take advantage of:
- Code reusability
- Support multiple data sources
- Abstraction and separation of concerns
- Maintainability and testability
In more complex scenarios, the generic repository pattern can limit flexibility and force you to run a simple query that retrieves all or just one. If you want to expand the query, you need to represent some ORM classes (e.g. DbContext) in the consumer, in our case the controller, and this undermines the benefit of separation of concerns and leads to a limitation of testability.
Implement Generic Repository Pattern C#
We implement the generic repository pattern C# by defining an interface contract that can handle CRUD operations for each entity type.
Setting Up the Project
Add a new Class library named Dnc.Staff.Repository
to the Staff
solution we created in the previous post Entity Framework Code First Approach in .NET 8. Select .NET 8 (Long Term Support) as the target Framework.
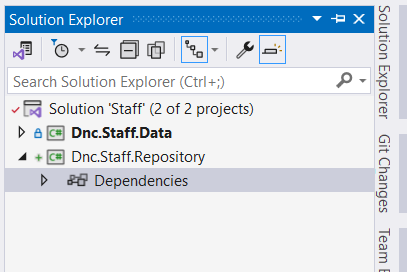
Second reference the Dnc.Staff.Data
project to the Dnc.Staff.Repository
project.
Defining the Generic Interface Contract
First create a new directory in the Dnc.Staff.Repository
project with the name Interfaces. Then add a new interface file namedIGenericRepository.cs
, as shown below.
namespace Dnc.Staff.Repository.Interfaces
{
public interface IGenericRepository<TEntity> where TEntity : class
{
Task<IEnumerable<TEntity>> GetAllAsync();
Task<IEnumerable<TEntity>> GetAllIncludeAsync(Expression<Func<TEntity, object>>[] properties);
Task<TEntity> FindByKey(int key);
Task<IEnumerable<TEntity>> FindByAsync(Expression<Func<TEntity, bool>> predicate);
Task<IEnumerable<TEntity>> GetByIncludeAsync(Expression<Func<TEntity, bool>> predicate, Expression<Func<TEntity, object>>[] properties);
Task<int> AddAsync(TEntity entity);
Task<int> AddRangeAsync(IEnumerable<TEntity> entities);
Task<int> UpdateAsync(TEntity entity);
Task<int> UpdateRangeAsync(IEnumerable<TEntity> entities);
Task<int> DeleteAsync(TEntity entity);
Task<int> DeleteRangeAsync(IEnumerable<TEntity> entities);
}
}
Implementing the Generic Repository
Create a new file class named GenericRepository.cs
in the Dnc.Staff.Repository
project.
The GenericRepository
follows the contract by implementing it , as shown in the following code.
namespace Dnc.Staff.Repository
{
public class GenericRepository<TEntity> : IGenericRepository<TEntity> where TEntity : class
{
public StaffDbContext Context { get; }
public DbSet<TEntity> Table { get; }
public GenericRepository(StaffDbContext context)
{
Context = context;
Table = Context.Set<TEntity>();
}
public async Task<IEnumerable<TEntity>> GetAllAsync()
{
return await Table.AsNoTracking().ToListAsync();
}
public async Task<IEnumerable<TEntity>> GetAllIncludeAsync(Expression<Func<TEntity, object>>[] properties)
{
var queryable = Table.AsNoTracking();
foreach (var property in properties)
{
queryable = queryable.Include(property);
}
return await queryable.ToListAsync();
}
public async Task<IEnumerable<TEntity>> GetByIncludeAsync(Expression<Func<TEntity, bool>> predicate, Expression<Func<TEntity, object>>[] properties)
{
var queryable = Table.AsNoTracking();
var query = properties.Aggregate(queryable, (current, property) => current.Include(property));
return await query.Where(predicate).ToListAsync();
}
public async Task<TEntity> FindByKey(int key)
{
return await Table.AsNoTracking().SingleOrDefaultAsync(BuildLambda<TEntity>(key));
}
public async Task<IEnumerable<TEntity>> FindByAsync(Expression<Func<TEntity, bool>> predicate)
{
return await Table.AsNoTracking().Where(predicate).ToListAsync();
}
public async Task<int> AddAsync(TEntity entity)
{
await Table.AddAsync(entity);
return await SaveChangesAsync();
}
public async Task<int> AddRangeAsync(IEnumerable<TEntity> entities)
{
await Table.AddRangeAsync(entities);
return await SaveChangesAsync();
}
public async Task<int> DeleteAsync(TEntity entity)
{
Table.Remove(entity);
return await SaveChangesAsync();
}
public async Task<int> DeleteRangeAsync(IEnumerable<TEntity> entities)
{
Table.RemoveRange(entities);
return await SaveChangesAsync();
}
public async Task<int> UpdateAsync(TEntity entity)
{
Table.Update(entity);
return await SaveChangesAsync();
}
public async Task<int> UpdateRangeAsync(IEnumerable<TEntity> entities)
{
Table.UpdateRange(entities);
return await SaveChangesAsync();
}
private static Expression<Func<TItem, bool>> BuildLambda<TItem>(int id)
{
var item = Expression.Parameter(typeof(TItem), "item");
var property = Expression.Property(item, "Id");
var constant = Expression.Constant(id);
var equal = Expression.Equal(property, constant);
return Expression.Lambda<Func<TItem, bool>>(equal, item);
}
private async Task<int> SaveChangesAsync()
{
try
{
return await Context.SaveChangesAsync();
}
catch (Exception ex)
{
throw new InvalidOperationException(ex.Message);
}
}
}
}
The GenericRepository
depends on the StaffDbContext
, and instead of hard-coding classes that you depend on into other classes, we push them in from somewhere else using Dependency Injection, which gives us more flexibility and loose coupling in the software and is beneficial when writing tests for these classes, as shown below.
public StaffDbContext Context { get; }
public DbSet<TEntity> Table { get; }
protected GenericRepository(StaffDbContext context)
{
Context = context;
Table = Context.Set<TEntity>();
}
The correct GenericRepository
instance is created by specifying the entity type to be used. The class then creates the correct DbSet
, which depends on the specified entity type, and the CRUD methods use this DbSet
to perform CRUD operations.
The FindByKey
method uses the lambda expression to determine the key, as you can see in the following code.
public async Task<TEntity> FindByKey(int key)
{
return await Table.AsNoTracking().SingleOrDefaultAsync(BuildLambda<TEntity>(key));
}
The BuildLambda
method creates dynamic LINQ queries with expression trees, which are a powerful tool for dynamic runtime behavior in .NET applications.
private static Expression<Func<TItem, bool>> BuildLambda<TItem>(int id)
{
var item = Expression.Parameter(typeof(TItem), "item");
var property = Expression.Property(item, "Id");
var constant = Expression.Constant(id);
var equal = Expression.Equal(property, constant);
return Expression.Lambda<Func<TItem, bool>>(equal, item);
}
The GetAllIncludeAsync
method uses eager loading to retrieve graphs. First a Queryable
is retrieved, then an Include method is built up for several navigation properties and then the ToListAsync
method is executed on the Queryable
result, as shown below.
public async Task<IEnumerable<TEntity>> GetAllIncludeAsync(Expression<Func<TEntity, object>>[] properties)
{
var queryable = Table.AsNoTracking();
foreach (var property in properties)
{
queryable = queryable.Include(property);
}
return await queryable.ToListAsync();
}
The GetByIncludeAsync
method is the same as the previous method, except that it takes a predicate for filtering Expression<Func<Tentity, bool>>
and only adds the filtering to get filtered graphs, as shown in the following code.
public async Task<IEnumerable<TEntity>> GetByIncludeAsync(Expression<Func<TEntity, bool>> predicate, Expression<Func<TEntity, object>>[] properties)
{
var queryable = Table.AsNoTracking();
var query = properties.Aggregate(queryable, (current, property) => current.Include(property));
return await query.Where(predicate).ToListAsync();
}
The remaining methods are self-explanatory and are simply implemented by the DbSet
methods.
Conclusion
To summarise, the Generic Repository Pattern C# is a powerful design pattern that simplifies data access by creating a single generic repository that can handle CRUD operations for each entity type instead of having a separate repository for each entity, which is really beneficial to take advantage of multiple data source support and code reusability.
Sample Code
You can find the complete sample code for this project on my GitHub repository
Enjoy This Blog?
Discover more from Dot Net Coder
Subscribe to get the latest posts sent to your email.