Table of Contents
Dependency injection in ASP.NET Core is a set of software design principles and patterns that enables you to remove dependency between objects by achieving the inversion of control (IoC) principle , this is one of the SOLID principles. DI makes it easy to create loosely coupled components.
What is Dependency injection in ASP.NET Core ?
Dependency injection (DI) is a technique in which an object or component receives objects that it depends on and consumes functionality defined by the dependency objects without having any knowledge of which implementation classes are being used.
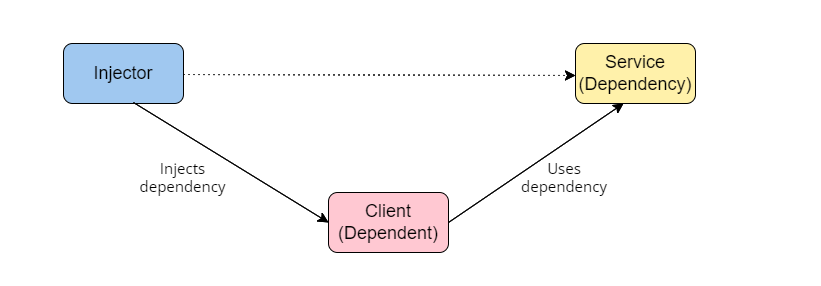
As the above diagram shows , the framework (Injector ) creates an object of dependency (Service) and injects it into the dependent component (Client), the Client consumes functionality defined by dependency to perform its operations
Different kinds of Dependency Injection
There are three main styles of dependency injection in which a client can receive injected services:
- Constructor Injection
- Setter Injection
- Method Injection
Constructor Injection
Constructor injection is the most common dependency injection type in which a class requests its dependencies through its constructor. The constructor injection is preferred when the class cannot do its function without the dependency or the dependency is used in multiple functions in the class
namespace DISampleWebAPI.Workflows
{
public class BookWorkflow : IBookWorkflow
{
private readonly IBookService bookService;
public BookWorkflow(IBookService bookService)
{
this.bookService = bookService;
}
public async Task<Book> GetBookById(int id)
{
return await bookService.GetBookById(id);
}
}
}
Setter Injection
In this kind of dependency injection, the class requests its dependencies through a setter method or a public property of the client class rather than a constructor, the Client uses the default implementation and gives the caller an option to set a different implementation as the following diagram shows.
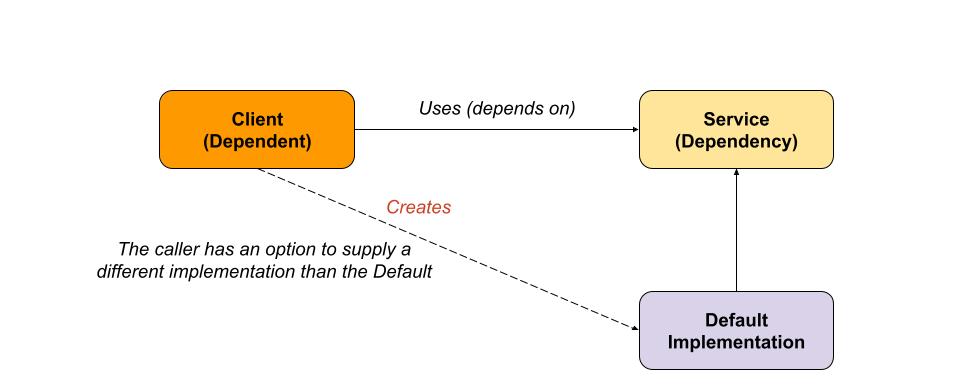
namespace DISampleWebAPI.Workflows
{
public class EmployeeWorkflow : IEmployeeWorkflow
{
private IEmployeeService employeeService;
public IEmployeeService EmployeeService
{
get => employeeService ?? throw
new InvalidOperationException("EmployeeService is not initialized");
set => employeeService = value;
}
public async Task<IEnumerable<Employee>> GetEmployees()
{
return await employeeService.GetEmployees();
}
}
}
Method Injection supplies the client (Dependent) with a dependency object by passing it as a method argument of a public method of the client class.
Use Method Injection when the Dependency varies on each call.
namespace DISampleWebAPI.Workflows
{
public class ProductWorkflow
{
public async Task<Product> GetProduct(int id, ProductService productService)
{
if (productService == null)
{
throw new ArgumentNullException(nameof(productService));
}
return await productService.GetProduct(id);
}
}
}
Dependency Injection (DI) in ASP.NET Core
.NET comes with a built-in IoC container framework as apart of the .Net framework witch is a great way to reduce coupling and increase testability . This comes with Microsoft.Extensions.DependencyInjection NuGet package.
There are two types of services in ASP.NET Core :
- Application Services: Services created by the developer.
- Framework Services: Services provided by the Framework such as IWebHostEnvironment, IConfiguration, etc.
Service Lifetimes
The IServiceProvider
implementation represents the The Built-in IoC that supports constructor injection by default.
ASP.NET Runtime creates all the required Framework Services and registers them with IoC container and allow us to register Application Services with a specified lifetime using the IServiceCollection instance which is available through the Services property of WebApplicationBuilder.
The Built-in IoC container manages the Application Services and disposes them automatically according to the specified lifetime.
Built-in IoC container in ASP.NET supports three types of lifetimes:
- Transient
- Singleton
- Scoped
Singleton
The IoC container create only one object of service throughout the application lifetime witch means the consumer gets the same object every time it is requested.
Transient
The IoC container returns a new object every time you ask for it, in this case the consumer gets a new instance every time it is requested. It is a safe choice but creates a lot of instances to be garbage collected.
Scoped
The IoC container creates an instance of service per request which means the instance will be shared by all the components that handle that request.
Let’s create an ASP.NET web API application to understand the service lifetime.
Also read https://dotnetcoder.com/azure-service-bus-integration-with-asp-net-core-web-api/
Service Lifetimes Example
Create a new ASP.NET Core web API application with the name Lifetime.Sample.WebApi
1. Create a new project folder with the name Services
Add three interface files with the names :
- IRandomService.cs
- IFirstService.cs
- ISecondService.cs
Add also three class files with the names :
- RandomService.cs
- FirstService.cs
- SecondService.cs
The IRandomService interface
The IRandomService interface is a simple interface with two methods.
namespace Lifetime.Sample.WebApi.Services
{
public interface IRandomService
{
int GetRandom();
void GenerateRandom();
}
}
The RandomService class
The RandomService class implements the IRandomService and generates a random number on the GetRandom call, and returns it on the GetRandom call.
namespace Lifetime.Sample.WebApi.Services
{
public class RandomService : IRandomService
{
private int random;
public void GenerateRandom() => random = new Random().Next(1,10);
public int GetRandom() => random;
}
}
The IFirstService, ISecondService interfaces
The two interfaces have the same methods.
public interface IFirstService
{
int Get();
void Genearet();
int GetRandomHashCode();
}
public interface ISecondService
{
int Get();
void Genearet();
int GetRandomHashCode();
}
The FirstService, SecondService classes
The FirstService class implements the IFirstService interface and depends on the IRandomService that is injected in its constructor.
The SecondService class in the same way implements the ISecondService interface and depends on the IRandomService that is injected in its constructor.
namespace Lifetime.Sample.WebApi.Services
{
public class FirstService : IFirstService
{
private readonly IRandomService randomService;
public FirstService(IRandomService randomService) => this.randomService = randomService;
public void Genearet() => randomService.GenerateRandom();
public int Get() => randomService.GetRandom();
public int GetRandomHashCode() => randomService.GetHashCode();
}
}
namespace Lifetime.Sample.WebApi.Services
{
public class SecondService : ISecondService
{
private readonly IRandomService randomService;
public SecondService(IRandomService randomService) => this.randomService = randomService;
public void Genearet() => randomService.GenerateRandom();
public int Get() => randomService.GetRandom();
public int GetRandomHashCode() => randomService.GetHashCode();
}
}
Both implementations have the ability to generate a random number and get the hashCode of the RandomService instance that is injected in the constructors.
2. Create RandomController
Create a new API controller with the name RandomController and modify the controller to get two instances of IFirstService and ISecondService through the constructor.
namespace Lifetime.Sample.WebApi.Controllers
{
[Route("api/[controller]")]
[ApiController]
public class RandomController : ControllerBase
{
private readonly IFirstService firstService;
private readonly ISecondService secondService;
public RandomController(IFirstService firstService, ISecondService secondService)
{
this.firstService = firstService;
this.secondService = secondService;
}
}
}
3. Create a Get method
Create a Get method, first we call the Generate method on the IFirstService instance.
The Method returns a Dictionary<string,object> that contains information about the instance used by the IFirstService and ISecondService for the three service lifetime.
namespace Lifetime.Sample.WebApi.Controllers
{
[Route("api/[controller]")]
[ApiController]
public class RandomController : ControllerBase
{
private readonly IFirstService firstService;
private readonly ISecondService secondService;
public RandomController(IFirstService firstService, ISecondService secondService)
{
this.firstService = firstService;
this.secondService = secondService;
}
[HttpGet]
public Dictionary<string,object> Get()
{
firstService.Genearet();
return new Dictionary<string, object>
{
["Random_Number_Service_1"] = firstService.Get(),
["Random_Number_Service_2"] = secondService.Get(),
["Instance_Hashcode_Service_1"] = firstService.GetRandomHashCode(),
["Instance_Hashcode_Service_2"] = secondService.GetRandomHashCode()
};
}
}
}
4. Registering the services in Program.cs
To register the services in Program.cs, use AddTransient extension method of IServiceCollection as shown in the following snippet:
builder.Services.AddTransient<IRandomService, RandomService>();
builder.Services.AddTransient<IFirstService, FirstService>();
builder.Services.AddTransient<ISecondService, SecondService>();
5. Running the application
Run the application with Transient service lifetime configuration, and observe the output
{
"Random_Number_Service_1": 8,
"Random_Number_Service_2": 0,
"Instance_Hashcode_Service_1": 23816093,
"Instance_Hashcode_Service_2": 54293426
}
Every time we call the API we see that the random numbers and the hash codes displayed for both instances of IRandomService are different.
This is because every time we call the API a new object for IRandomService is created, which means every time the IFirstService and ISecondService request an object of the IRandomService, the IoC container will create a new instance.
Modify the service lifetime of the IRandomService from Transient to Singleton
builder.Services.AddSingleton<IRandomService, RandomService>();
builder.Services.AddTransient<IFirstService, FirstService>();
builder.Services.AddTransient<ISecondService, SecondService>();
Run the application with Singleton service lifetime setup, and observe the output
{
"Random_Number_Service_1": 5,
"Random_Number_Service_2": 5,
"Instance_Hashcode_Service_1": 3686381,
"Instance_Hashcode_Service_2": 3686381
}
The hash codes and the random numbers of IRandomService in both IFirstService and ISecondService are the same.
Every time you call the API you will see that the hash codes don’t change but the random number changes and it is shared, this is because the IoC container creates only one object of IRandomService throughout the application lifetime and shares it between the IFirstService and ISecondService.
Modify the service lifetime of the IRandomService from Singleton to Scoped
builder.Services.AddScoped<IRandomService, RandomService>();
builder.Services.AddTransient<IFirstService, FirstService>();
builder.Services.AddTransient<ISecondService, SecondService>();
Run the application with Scoped service lifetime setup, and observe the output
{
"Random_Number_Service_1": 9,
"Random_Number_Service_2": 9,
"Instance_Hashcode_Service_1": 51781231,
"Instance_Hashcode_Service_2": 51781231
}
The hash codes and the random numbers for both instances are the same and they update on every call, This is because only one instance for IRandomService is created per request scope.
Conclusion
In this post, we have explained the DI concept that enables you to remove the dependency between objects and create more loosely coupled components
We described the types of dependency injection and the different ways that services are consumed, we have also explained how IoC container manages the object lifetime with different types of registrations.
The code for the demo can be found Here
Also read https://dotnetcoder.com/datagrid-blazor-with-sorting-and-filtering/
Enjoy This Blog?
Discover more from Dot Net Coder
Subscribe to get the latest posts sent to your email.