Table of Contents
Authorization Code Flow Azure Ad Example
Take a look at the finished Authorization Code Flow Azure Ad example implemented in .Net Core and Azure Ad ( Entra ID).
What is Authorization Code Flow?
The authorization code flow is an OAuth 2.0 mechanism and one of the most frequently used flows. It consists of a two-step approach to obtain tokens. In the first step, a so-called authorization code is returned. In the second step, the client receives a set of tokens, including an access token, by securely exchanging the authorization code for an access token on the backend server.
When to use Authorization Code Flow?
The following list contains some general requirements for selecting the Authorization Code Flow in your application.
- The client application is deployed on a web server and the code is not made publicly available.
- The client application has a backend server that can securely handle secret keys (e.g. storage of the client ID and the client secret).
- The client application must be integrated with external APIs (e.g. Google APIs).
If your application is a single-page SPA application, such as a mobile and JavaScript-based application that has no backend, you should use Authorization Code Flow with PKCE, which provides an additional layer for better security. We will talk about Authorization Code Flow with PKCE in later sections.
Authorization Code Flow Diagram
The following diagram shows the authorization code flow in detail.
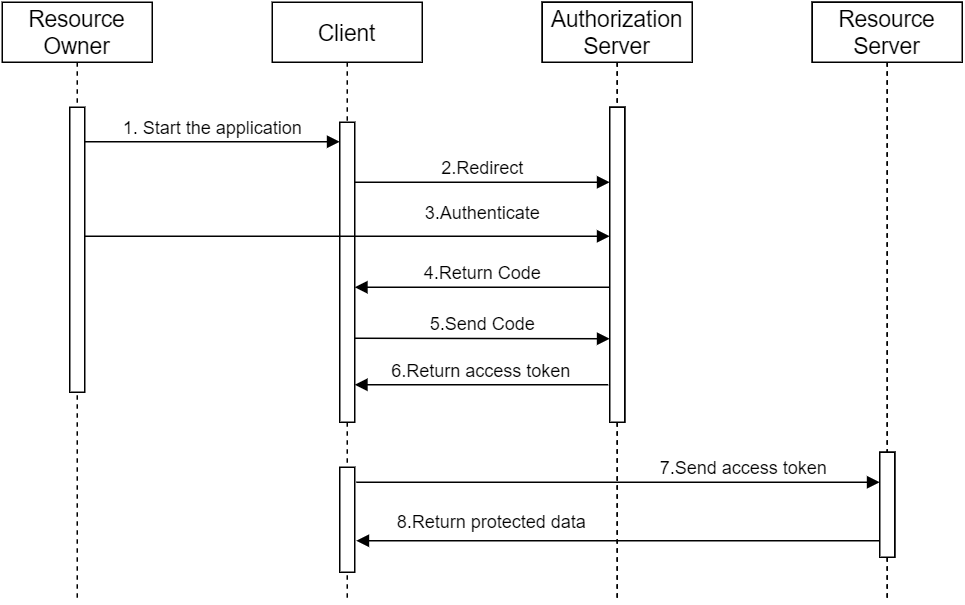
The diagram above shows how the authorization code flow works.
The Authorization code consists of several steps. In the first step, the client starts the flow on behalf of the user and in the next step, the Authorization server returns a so-called authorization code, which is used in the last step by exchanging the authorization code for a set of tokens, including the access token, which we can send to a resource server (API).
Implementing Authorization Code Flow Azure Ad
In the previous section we have discovered the basic concepts of Authorization Code flow. In this section, we will implement Authorization Code Flow Azure Ad by creating a solution that contains two projects and then we will register them in the Azure Active Directory ( Entra ID).
The first project is an ASP.NET Core Web API that is secure with Microsoft Identity, and the second is a console application that pretends to be a web application.
Setting up the project
To implement Authorization Code Flow Azure Ad in practice , open Visual Studio and create a blank solution named AuthorizationCodeFlow
.
Then add a new ASP.NET Core Web API project template named Dnc.WebApi
and select .NET 8 (Long Term Support) as the target Framework. and then add a new console application named Dnc.ConsoleClient
to the AuthorizationCodeFlow
solution pretending to be a web application.
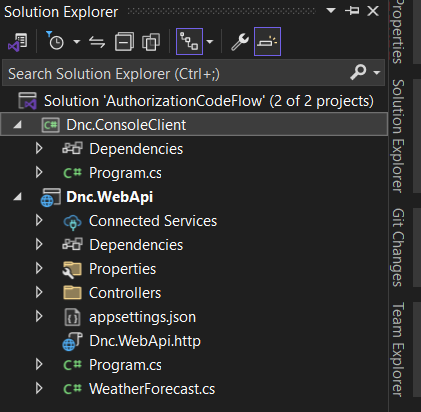
Registering the Web API in Microsoft Entra ID (Azure Ad)
You need An Azure account, subscription, go to the Azure Portal .
Go to Azure Active Directory “Entra ID” in the left navigation pane and click “App Registrations” and then click “New Registration” and finally enter the following information as shown below.
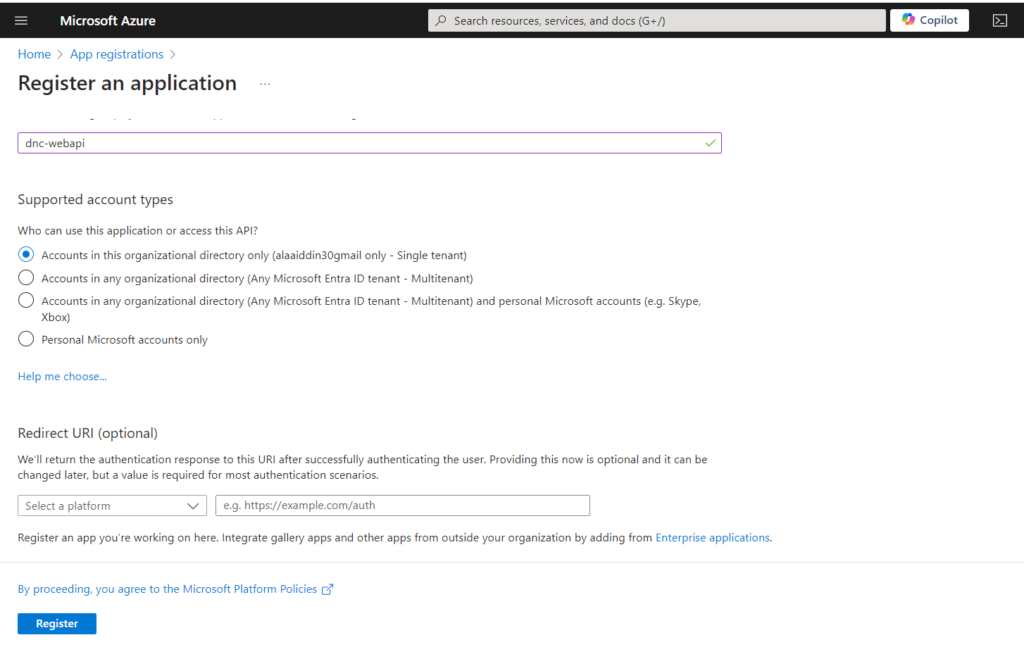
Click Register.
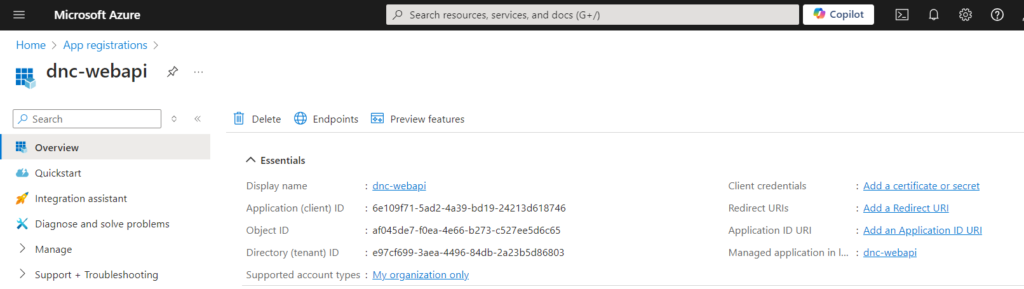
We now need to expose our API to make it consumable.
Go to “Expose an API”, set the Application ID URI (use something like api://{clientId}
) , I will use my domain name/dnc-webapi
, and click “Add a scope” and enter the required information and click “Add scope” as shown below.
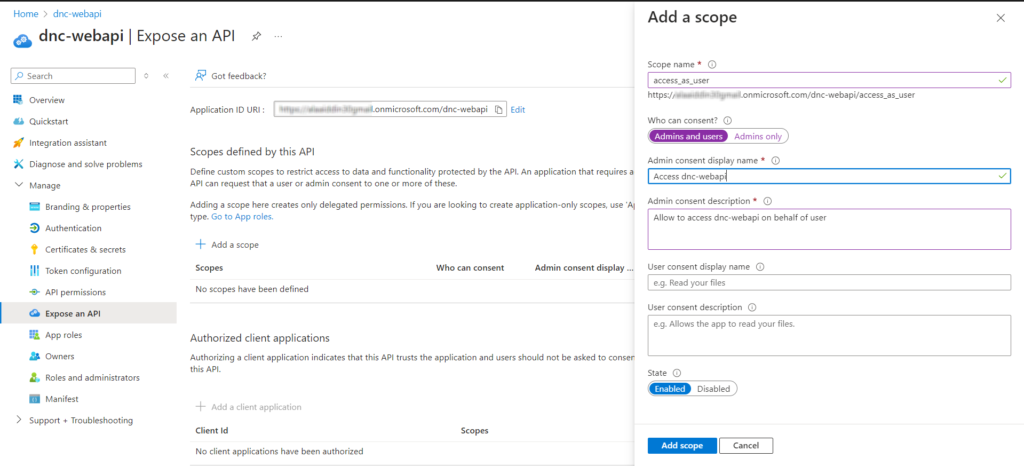
We have exposed our API by adding a scope named “access_as_user”.
The scope defines the level of access that an application requests when interacting with APIs. In simple words, APIs can expose multiple things that a user or application can do.
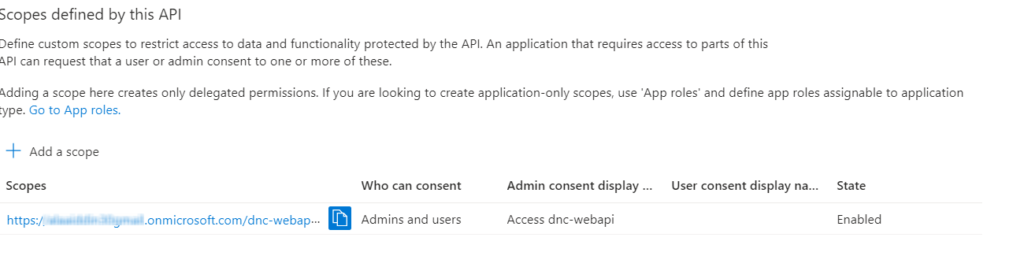
Registering the Console App in Microsoft Entra ID (Azure Ad)
Go to Azure Active Directory “Entra ID” in the left navigation pane and click “App Registrations” and then click “New Registration” and finally enter the following information as shown below.
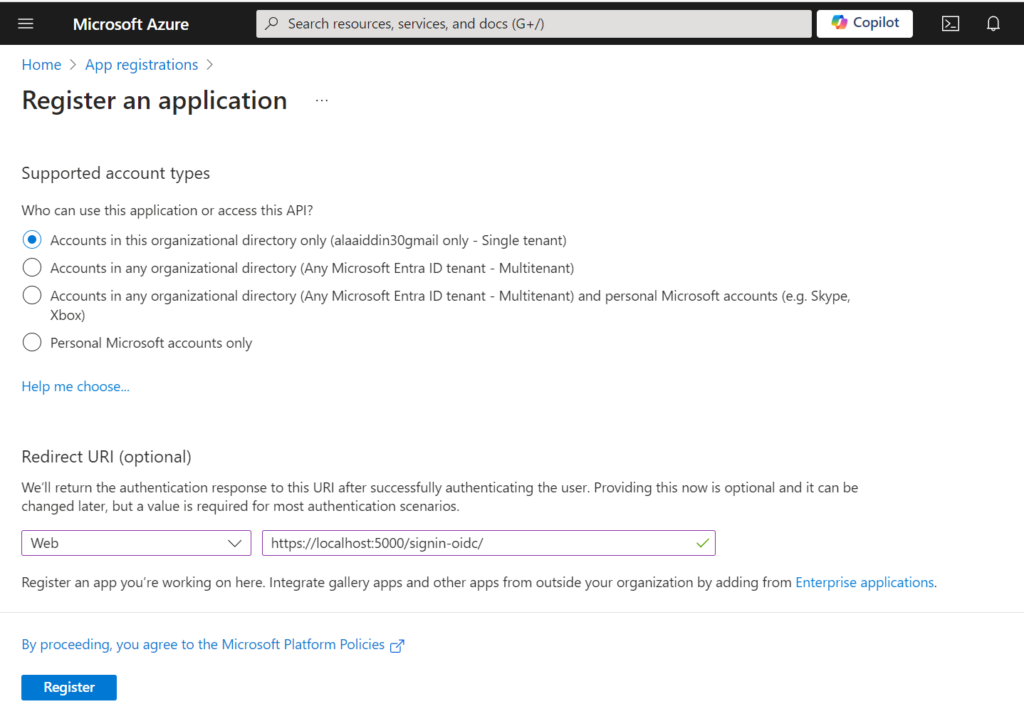
Click on “Register” and copy the required information for later use.
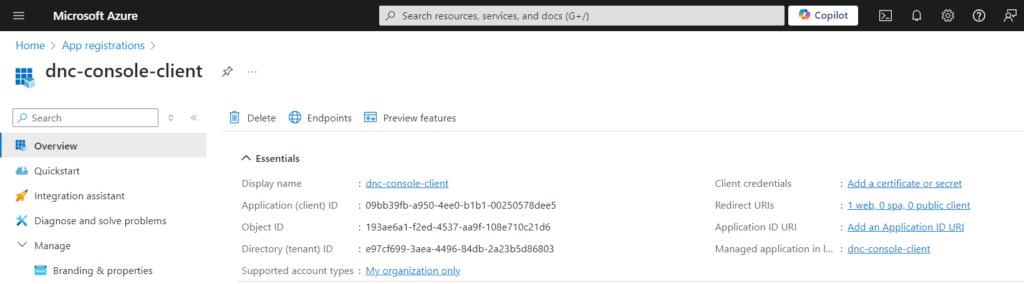
Second: Go to “Certificate and secrets” , then to “New Client Secret” and enter the required information and then click “Add” as shown below.
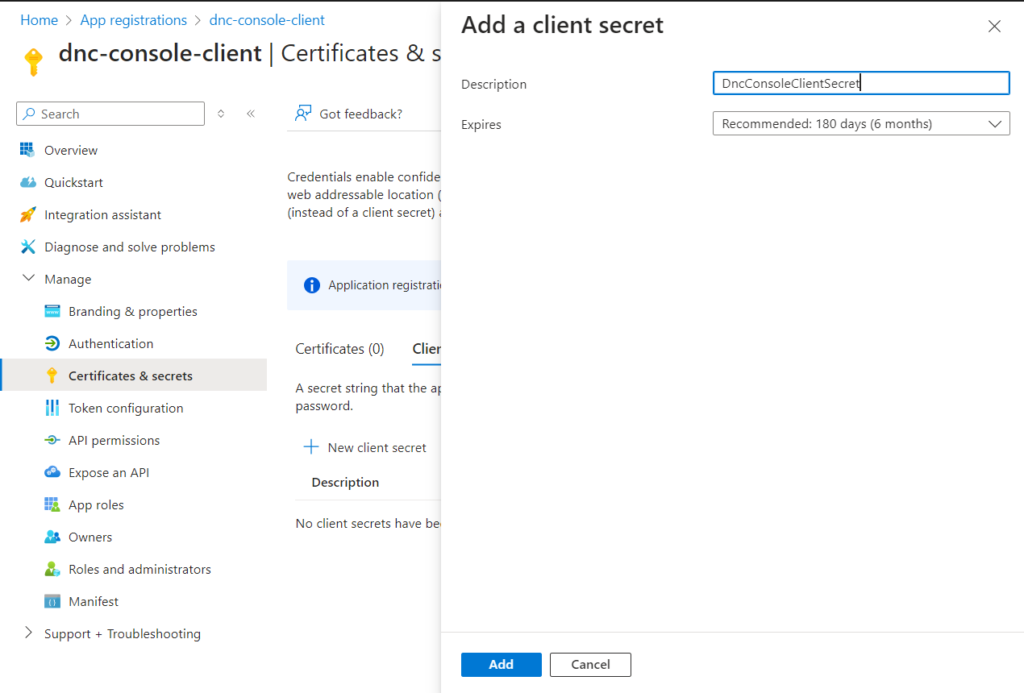
Copy the key and save it in a safe place. This key is no longer visible after you have closed the tile.
Our client application must have a permission to call the ASP.NET Core API registered in the previous section.
Go to “API Permissions” and then to “Add a permission”. Select the access_as_user
permission and click “Add permissions” as shown below.
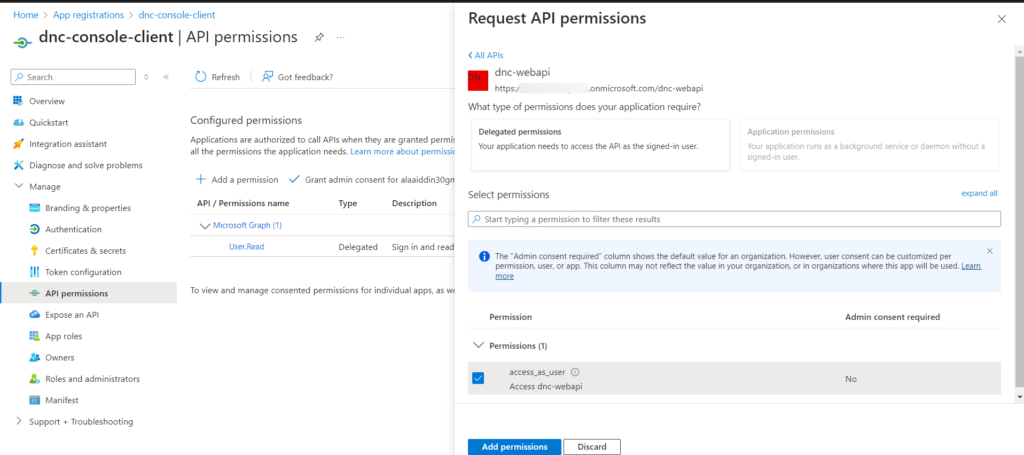
finally: Grant admin consent by clicking on the “Grant Admin Consent” button.
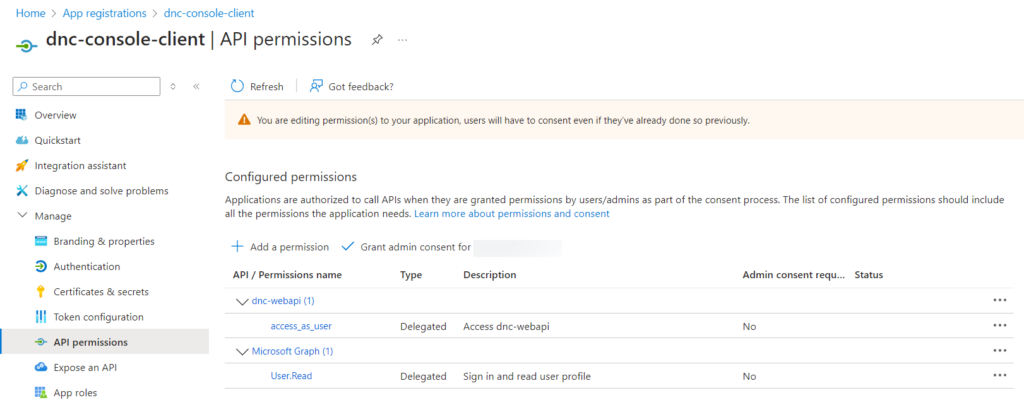
Configuring the Web API
To secure our ASP.NET Core Web API 8 with Azure Active Directory (Entra ID), we first need to configure our Azure AD credentials in the appsettings.json
file as shown below.
{
"AzureAd": {
"Instance": "https://login.microsoftonline.com/",
"Domain": "YOUR DOMAIN",
"TenantId": "e97cf699-3aea-4496-84db-2a23b5d86803",
"ClientId": "6e109f71-5ad2-4a39-bd19-24213d618746",
"Audience": "YOUR DOMAIN/dnc-webapi"
}
}
Avoid storing security information in your code. It is recommended to securely manage the secret by using Azure Key Vault instead of embedding it directly in your code.
Second, add the required NuGet packages with the following commands.
dotnet add package Microsoft.Identity.Web
dotnet add package Microsoft.AspNetCore.Authentication.JwtBearer
Finally, update the Program.cs
file by adding the following code.
// Removed code for brevity
builder.Services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme)
.AddMicrosoftIdentityWebApi(builder.Configuration.GetSection("AzureAd"));
builder.Services.AddAuthorizationBuilder()
.AddPolicy("access_as_user", policy =>
policy.RequireScope("access_as_user"));
var app = builder.Build();
builder.Services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme)
: tells the Web API to use JWT bearer token for authentication.
.AddMicrosoftIdentityWebApi(builder.Configuration.GetSection("AzureAd"))
: adds Microsoft Identity Platform as the authentication provider.
We have also defined a custom policy to protect our routes, which can be used in our controller at the controller or action level, as shown below.
namespace Dnc.WebApi.Controllers
{
[ApiController]
[Route("[controller]")]
public class WeatherForecastController : ControllerBase
{
[Authorize(Policy = "access_as_user")]
[HttpGet]
public IEnumerable<WeatherForecast> Get()
{
// Removed code for brevity
}
}
}
Set the Dnc.WebApi
as Startup project and observe the result (401 status code) when you call the protected route.
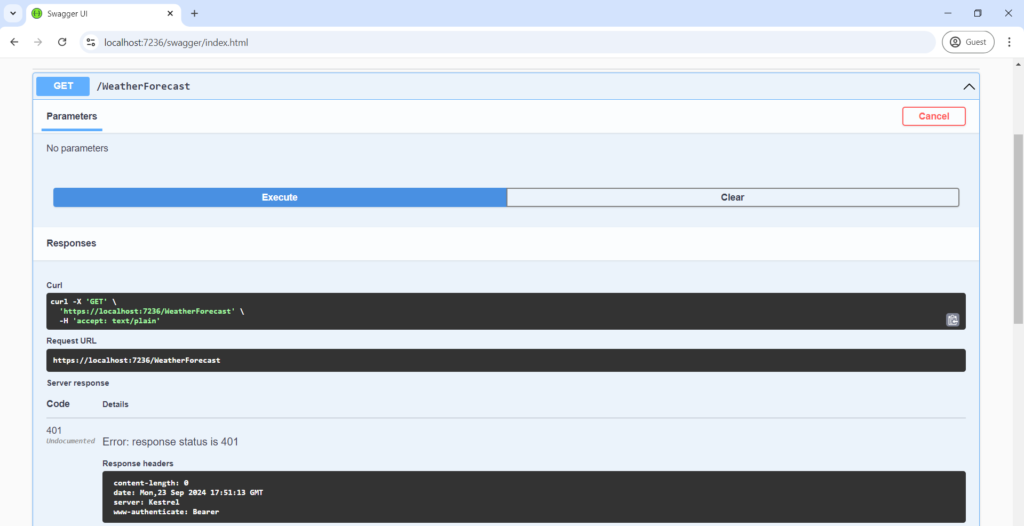
Getting the authorization code
The Azure configurations are ready for both console client and Web API is secure with Microsoft Management Identity.
The next step is to configure our client to call the secure ASP.NET Core Web API 8 with Azure Active Directory (Entra ID).
To better understand the code flow, it can be helpful to know the basic form of HTTP messages. The client (in our case the console application) creates an HTTP GET authorization request and the browser sends it to the Authorization server
The authorization request contains parameters that tell the Authorization server what it should return response_type=code
and the redirect_uri
parameter that informs the authorization server where to return the authorization code. We should explicitly specify the redirect URI, as the Authorization server will only return the authorization code if it trusts the redirect URI.
GET https://login.microsoftonline.com/e97cf699-3aea-4496-84db-7a23b5d89803/oauth2/v2.0/authorize
?client_id=clientId
&redirect_uri=https://www.example.com/callback
&response_type=code
&scope=access_as_user
&code_challenge=aB3f8Gh9Kl_aB3f8Gk9Kh
&code_challenge_method=S256
We have configured client_id
, redirect_uri
and scope
parameters for our client in Azure Ad.
Also read https://dotnetcoder.com/on-behalf-of-flow-entra-id/
Proof Key for Code Exchange (PKCE)
The Proof Key for Code Exchange is an additional security measure in the OAuth 2.0 Authorization Code Flow that allows the authorization server to correlate an authorization request and a token request. The authorization server can verify that an authorization request and a token request are part of the same flow by associating the authorization code with a challenge.
Let’s create a method in our console app that returns an authorization code in Authorization code flow Azure Ad.
In .NET console apps, I have added the appsettings.json
file that contains the secrets and other configuration keys, but you can hard-code them into your method if you want.
{
"AzureAd": {
"Instance": "https://login.microsoftonline.com",
"Domain": "YOUR DOMAIN",
"TenantId": "e97cf699-3aea-4496-84db-2a23b5d86803",
"ClientId": "09bb39fb-a950-4ee0-b1b1-00250578dee5",
"ClientSecret": "rGU8Q~oK7ecKPgfI~fYN.BVD-r~NlibK3wHe3cJ5",
"RedirectUri": "http://localhost:5000/signin-oidc/",
"ApplicationId": "https://YOUR DOMAIN/dnc-webapi",
"Scopes": "access_as_user"
}
Update the Program.cs
as shown below.
namespace Dnc.ConsoleClient
{
internal class Program
{
static async Task Main(string[] args)
{
var configuration = new ConfigurationBuilder()
.SetBasePath(Directory.GetCurrentDirectory())
.AddJsonFile("appsettings.json", optional: false, reloadOnChange: true)
.Build();
Config.Configuration = configuration;
Console.WriteLine("Press Enter to begin");
Console.ReadLine();
var code = await GetAuthorizationCodeAsync();
Console.ForegroundColor = ConsoleColor.Cyan;
Console.WriteLine("Press Enter to display the Authorization Code");
Console.ReadLine();
Console.WriteLine($"************Authorization Code***************");
Console.WriteLine($"code: {code}");
}
static async Task<string> GetAuthorizationCodeAsync()
{
var tenantId = Config.Configuration["AzureAd:TenantId"];
var clientId = Config.Configuration["AzureAd:clientId"];
var redirectUri = Config.Configuration["AzureAd:RedirectUri"];
var instance = Config.Configuration["AzureAd:Instance"];
var responseType = "code";
var scopes = "openid";
var codeVerifier = "MyRandomCodeVerifier"; // Must be generated
var hash = System.Security.Cryptography.SHA256.HashData(System.Text.Encoding.UTF8.GetBytes(codeVerifier));
var codeChallenge = Convert.ToBase64String(hash)
.TrimEnd('=')
.Replace('+', '*')
.Replace('/', '_');
var codeEndpoint = $"{instance}/{tenantId}/oauth2/v2.0/authorize";
var authorizationUrl = $"{codeEndpoint}?response_type={responseType}&client_id={clientId}&redirect_uri={redirectUri}&scope={scopes}&code_challenge={codeChallenge}&code_challenge_method=S256";
Process.Start(new ProcessStartInfo
{
FileName = authorizationUrl,
UseShellExecute = true
});
using var listener = new HttpListener();
listener.Prefixes.Add(redirectUri);
try
{
listener.Start();
var context = await listener.GetContextAsync();
var authorizationCode = context.Request.QueryString["code"];
listener.Stop();
return authorizationCode;
}
catch (Exception ex)
{
Console.WriteLine(ex.Message);
throw;
}
}
}
public static class Config
{
public static IConfiguration Configuration { get; set; }
}
}
The GetAuthorizationCodeAsync
method starts a new process to open the URI with the default browser in the system with the required parameters in the authorization request, the client also calculates a hash via the code verifier and converts it to a string. We use the HttpListener
class to simulate a web application. It is a simple server that listens for the incoming request from the authorization server Azure Entra ID.
Run the Dnc.ConsoleClient
, enter your credentials and watch the console.
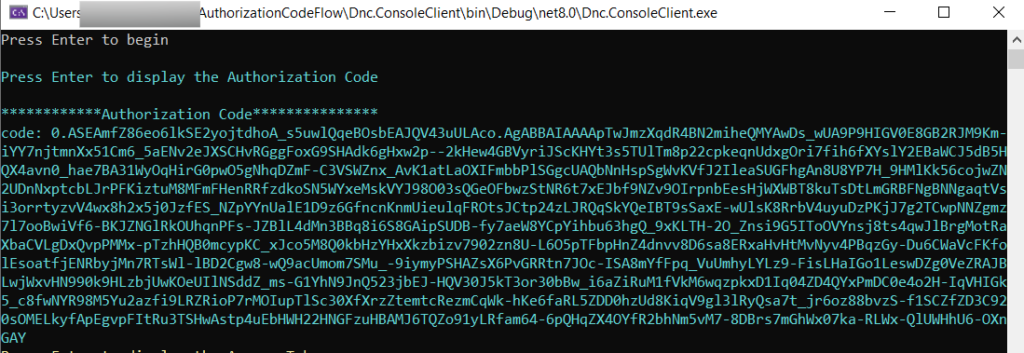
Exchanging the Authorization Code for an access token
In the previous section, we created a method to retrieve the authorization code from the Authorization server (Azure Ad) using Authorization code flow Azure Ad. If the authorization response is successful, the client sends the authorization code to the token endpoint of the authorization server. The client (in our case, the console application) should specify certain parameters grant_type=authorization_code
indicates that the client is using the authorization code flow to obtain an access token, and the client must specify its credentials client_id
and client_secret
, scope
(e.g. API permissions), as shown in the following code snippet.
POST https://login.microsoftonline.com/e97cf699-3aea-4496-84db-7a23b5d89803/oauth2/v2.0/token
Content-Type: application/x-www-form-urlencoded
Accept: application/json
Authorization: Basic h2VimjkWNsaWVlludDpVlkgdsxfhj=
code= ASEAmfZ86eo6lkSE2yojtdhoA_s5uwlQqeBOsbEAJQV43uULAco
&grant_type=authorization_code
&redirect_uri=http://localhost:5000/signin-oidc/
&code_verifier=ZBlL4dMn3BBq8i6S8GAipSUDB-fy7aeW8YCpYihbu63hgQ_9xK7T
Update the Program.cs
as shown below.
namespace Dnc.ConsoleClient
{
internal class Program
{
static async Task Main(string[] args)
{
// Removed code for brevity
var tokens = await ExchangeAutCodeForAccessToken(code);
var accessToken = tokens["access_token"];
Console.WriteLine("Press Enter to display the Access Token");
Console.ReadLine();
Console.WriteLine($"************Access Token***************");
Console.WriteLine($"{accessToken}");
}
static async Task<Dictionary<string, string>> ExchangeAutCodeForAccessToken(string authorizationCode)
{
var instance = Config.Configuration["AzureAd:Instance"];
var tenantId = Config.Configuration["AzureAd:tenantId"];
var applicationId = Config.Configuration["AzureAd:ApplicationId"];
var clientId = Config.Configuration["AzureAd:clientId"];
var clientSecret = Config.Configuration["AzureAd:ClientSecret"];
var redirectUri = Config.Configuration["AzureAd:RedirectUri"];
var scopes = Config.Configuration["AzureAd:Scopes"];
var scope = $"{applicationId}/{scopes}";
var grantType = "authorization_code";
var codeVerifier = "MyRandomCodeVerifier";
var tokenEndpoint = $"{instance}/{tenantId}/oauth2/v2.0/token";
var parameters = new Dictionary<string, string>
{
{ "grant_type", grantType },
{ "client_id", clientId },
{ "client_secret", clientSecret},
{ "code", authorizationCode },
{ "redirect_uri", redirectUri },
{ "scope", scope },
{ "code_verifier", codeVerifier }
};
var encodedContent = new FormUrlEncodedContent(parameters);
using var client = new HttpClient();
var response = await client.PostAsync(tokenEndpoint, encodedContent);
var test = await response.Content.ReadAsStringAsync();
if (!response.IsSuccessStatusCode)
throw new HttpRequestException($"Request failed with status code {response.StatusCode}");
using var doc = JsonDocument.Parse(await response.Content.ReadAsStringAsync());
return new Dictionary<string, string>
{
{
"access_token", doc.RootElement.TryGetProperty("access_token", out var access_token) ?
access_token.GetString() : throw new InvalidOperationException("access token not found.")
}
};
}
}
}
ExchangeAutCodeForAccessToken
uses the HttpClient
and sends a POST
request to the token endpoint of the authorization server with the required parameters to exchange the authorization code for access token.
Run the Dnc.ConsoleClient
and observe the access token as shown below.
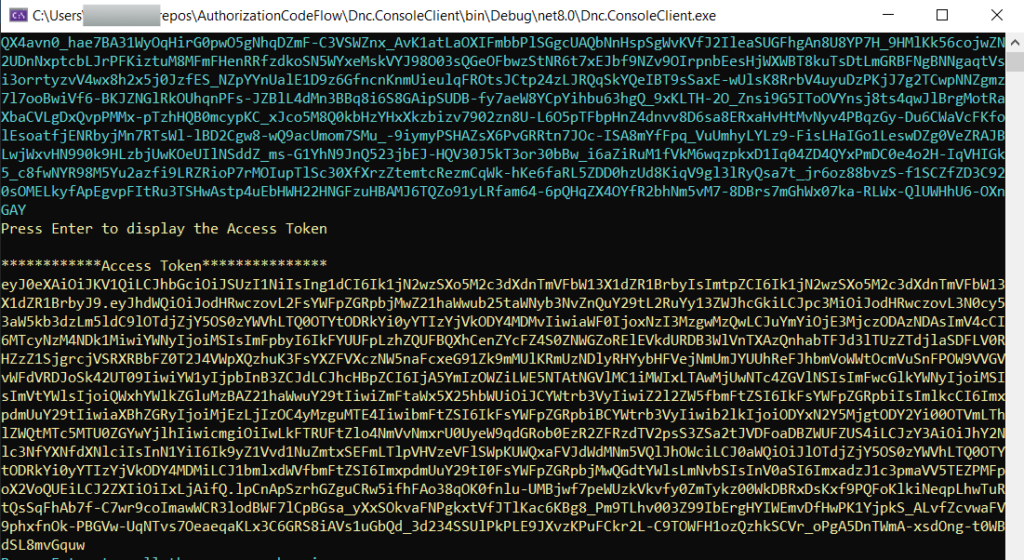
When a client in the Authorization code flow Azure Ad receives an access_token
, the authorization server in the Authorization code flow Azure Ad can return a refresh_token
along with the access_token
by including the offline_access
built into scope in the token request, since the access_token
is short-lived and generally expires within an hour and the client must receive a new access_token
. The client can use the refresh token to renew an expired access token without the user having to re-authenticate.
Update the GetAuthorizationCodeAsync
to return the refresh_token
by adding the offline_access
scope to the token request.
namespace Dnc.ConsoleClient
{
internal class Program
{
//Removed code for brevity
static async Task Main(string[] args)
{
var refreshToken = tokens["refresh_token"];
Console.WriteLine("Press Enter to display the refresh Token");
Console.ReadLine();
Console.WriteLine($"************Refresh Token***************");
Console.WriteLine($"{refreshToken}");
}
static async Task<Dictionary<string, string>> ExchangeAutCodeForAccessToken(string authorizationCode)
{
var instance = Config.Configuration["AzureAd:Instance"];
var tenantId = Config.Configuration["AzureAd:tenantId"];
var applicationId = Config.Configuration["AzureAd:ApplicationId"];
var clientId = Config.Configuration["AzureAd:clientId"];
var clientSecret = Config.Configuration["AzureAd:ClientSecret"];
var redirectUri = Config.Configuration["AzureAd:RedirectUri"];
var scopes = Config.Configuration["AzureAd:Scopes"];
var scope = $"{applicationId}/{scopes} offline_access";
var grantType = "authorization_code";
var codeVerifier = "MyRandomCodeVerifier";
var tokenEndpoint = $"{instance}/{tenantId}/oauth2/v2.0/token";
var parameters = new Dictionary<string, string>
{
{ "grant_type", grantType },
{ "client_id", clientId },
{ "client_secret", clientSecret},
{ "code", authorizationCode },
{ "redirect_uri", redirectUri },
{ "scope", scope },
{ "code_verifier", codeVerifier }
};
var encodedContent = new FormUrlEncodedContent(parameters);
using var client = new HttpClient();
var response = await client.PostAsync(tokenEndpoint, encodedContent);
var test = await response.Content.ReadAsStringAsync();
if (!response.IsSuccessStatusCode)
throw new HttpRequestException($"Request failed with status code {response.StatusCode}");
using var doc = JsonDocument.Parse(await response.Content.ReadAsStringAsync());
return new Dictionary<string, string>
{
{
"access_token", doc.RootElement.TryGetProperty("access_token", out var access_token) ?
access_token.GetString() : throw new InvalidOperationException("access token not found.")
},
{
"refresh_token", doc.RootElement.TryGetProperty("refresh_token", out var refresh_token) ?
refresh_token.GetString() : throw new InvalidOperationException("refresh token not found.")
}
};
}
}
}
Our GetAuthorizationCodeAsync method now returns a dictionary that contains both access_token
and refresh_token
.
Run the Dnc.ConsoleClient
again and observe the refresh token as shown below.
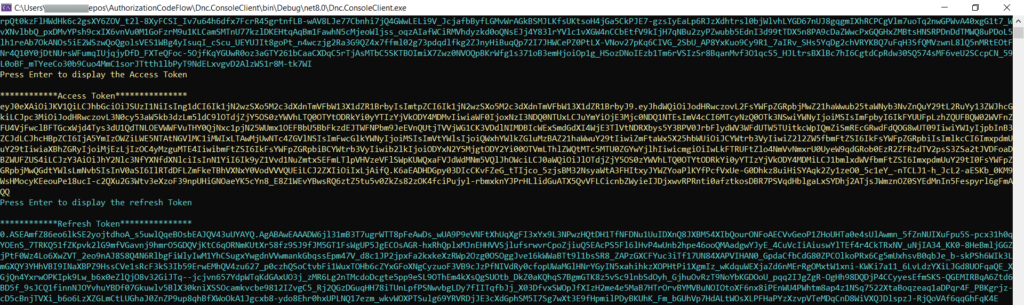
Calling the secure API
With the access token in hand, we can finally call our secure API by inserting the access token into the Authorization header, as shown in the following method.
static async Task<string> GetWeatherForecast(string accessToken)
{
using var httpClient = new HttpClient { BaseAddress = new Uri("https://localhost:7236/") };
httpClient.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", accessToken);
return await httpClient.GetStringAsync("WeatherForecast");
}
Update the Program.cs
as shown below.
namespace Dnc.ConsoleClient
{
internal class Program
{
static async Task Main(string[] args)
{
// Removed code for brevity
var data= await GetWeatherForecast(accessToken);
Console.WriteLine("Press Enter to call the secure web api");
Console.ReadLine();
Console.WriteLine($"************Response from the API***************");
Console.WriteLine($"{data}");
}
static async Task<string> GetWeatherForecast(string accessToken)
{
using var httpClient = new HttpClient { BaseAddress = new Uri("https://localhost:7236/") };
httpClient.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", accessToken);
return await httpClient.GetStringAsync("WeatherForecast");
}
}
}
We need to run multiple projects simultaneously in our Visual Studio 2022. To do this, select Properties and then Multiple Startup Projects in the solution and set the action for both projects to Start and press F5 for lunch.
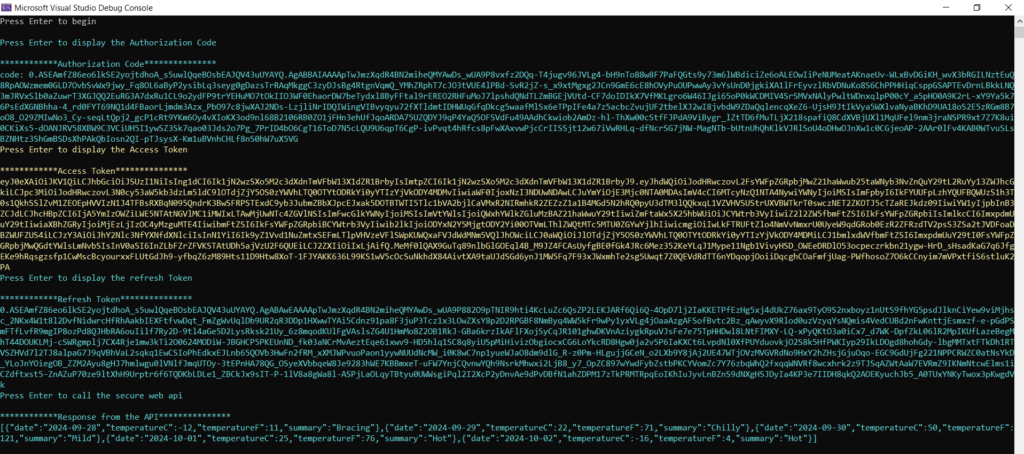
Conclusion
In this article, we gave an overview of the authorization code flow with PKCE and then implemented the Azure Ad authorization code flow Azure Ad with minimal code. We used the authorization code flow with PKCE to secure the code flow and mitigate common attacks.
Also read https://dotnetcoder.com/client-credentials-flow-in-entra-id/
Sample code
You can find the complete example code for this project on my GitHub repository
Enjoy This Blog?
Discover more from Dot Net Coder
Subscribe to get the latest posts sent to your email.