Table of Contents
Introduction
The Select element is a commonly used UI element in web development. By building a reusable Blazor select component, you can significantly save time and effort on future projects.
Take a look at the finished Blazor select component implemented in a Blazor Web App.
<DncSelect @bind-Value="@MyEmployee.Age">
<DncOption Value="default(int)" IsDefaultOption>Select Age</DncOption>
<DncOption Value="30" />
<DncOption Value="40" />
<DncOption Value="50" Disabled />
</DncSelect>
In the previous post, we created a Blazor Dropdown List Component in a slightly different way.
Why Use Reusable Components?
Building a reusable Blazor select component is an efficient way to leverage the power of Blazor’s framework for creating reusable components. As a commonly used UI element in web development, the Select element plays a crucial role. By investing time and effort in building this component, you can streamline future projects and save valuable resources
Setting Up the Project
Open Visual Studio and create a blank solution named Select
. Add a new Razor Class library named Dnc.Common.Razor
to the solution. Select .NET 8 (Long Term Support) as the target Framework.
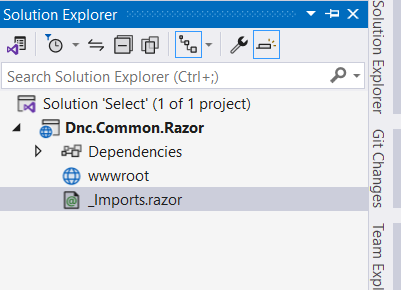
Creating the DncSelect component
First, create a new folder in the Dnc.Common.Razor
project named Select. Then, create a new class file called DncSelect
, and identify it as a generic class with a single generic parameter called TValue
, and add the following code.
@using System.Diagnostics.CodeAnalysis
@using System.Linq.Expressions
@using System.Globalization
@using Microsoft.AspNetCore.Components.Forms
@inherits InputBase<TValue>
@typeparam TValue
<select class="form-select" @bind="SelectedValue">
@ChildContent
</select>
@code {
[Parameter]
public RenderFragment ChildContent { get; set; }
[Parameter]
public Func<string, TValue> TypeConverter { get; set; }
[Parameter]
public CultureInfo CultureInfo { get; set; }
public string SelectedValue
{
get { return CurrentValueAsString; }
set { CurrentValueAsString = value; }
}
protected override bool TryParseValueFromString(string value,
[MaybeNullWhen(false)] out TValue result, [NotNullWhen(false)] out string validationErrorMessage)
{
try
{
if (TypeConverter != null)
{
result = TypeConverter(value);
}
else
{
if (CultureInfo != null)
{
result = DncConverter.ChangeType<TValue>(value, CultureInfo);
}
else
{
result = DncConverter.ChangeType<TValue>(value);
}
}
validationErrorMessage = null;
return true;
}
catch (Exception ex)
{
result = default(TValue);
validationErrorMessage = ex.Message;
return false;
}
}
}
We have defined a RenderFragment
property in DncSelect
that allows us to inject the embedded content into the component.
<select class="form-select" @bind="SelectedValue">
@ChildContent
</select>
The CurrentValueAsString
provided by the InputBase
class is used to get and set the SelectedValue
of the control , as you see below.
public string SelectedValue
{
get { return CurrentValueAsString; }
set { CurrentValueAsString = value; }
}
Since the DncSelect
class inherits from the InputBase<TValue>
generic abstract class for Blazor controls, we need to override the TryParseValueFromString
method, which is called whenever the value of the control changes.
TheTypeConverter
parameter is used to convert the result output parameter of the TryParseValueFromString
method to the TValue
type , or we use the custom converter DncConverter
if the TypeConverter
is null, which will be created later .
protected override bool TryParseValueFromString(string value,
[MaybeNullWhen(false)] out TValue result, [NotNullWhen(false)] out string validationErrorMessage)
{
try
{
if (TypeConverter != null)
{
result = TypeConverter(value);
}
else
{
if (CultureInfo != null)
{
result = DncConverter.ChangeType<TValue>(value, CultureInfo);
}
else
{
result = DncConverter.ChangeType<TValue>(value);
}
}
validationErrorMessage = null;
return true;
}
catch (Exception ex)
{
result = default(TValue);
validationErrorMessage = ex.Message;
return false;
}
}
Creating the DncConverter
TheTypeConverter
parameter is used to convert the result output parameter of the TryParseValueFromString
method to the TValue
type if the TypeConverter
is null, or it throws an InvalidCastException
exception if the conversion fails.
namespace Dnc.Common.Razor.Select
{
public static class DncConverter
{
public static TValue ChangeType<TValue>(string value, CultureInfo cultureInfo)
{
if (string.IsNullOrEmpty(value))
{
return default;
}
Type targetType = typeof(TValue);
try
{
if (targetType == typeof(string))
{
return (TValue)(object)value;
}
if (targetType == typeof(Guid))
{
return (TValue)(object)new Guid(value);
}
if (Nullable.GetUnderlyingType(targetType) is Type underlyingType)
{
targetType = underlyingType;
}
if (targetType == typeof(DateTime))
{
return (TValue)(object)DateTime.Parse(value, cultureInfo);
}
if (targetType == typeof(bool))
{
return (TValue)(object)bool.Parse(value);
}
if (targetType.IsEnum)
{
return (TValue)Enum.Parse(targetType, value);
}
if (targetType.IsValueType || targetType.IsPrimitive)
{
return (TValue)Convert.ChangeType(value, targetType, cultureInfo);
}
throw new InvalidCastException($"Cannot convert '{value}' to {targetType.Name}.");
}
catch (Exception ex)
{
throw new InvalidCastException($"Cannot convert '{value}' to {targetType.Name}.", ex);
}
}
public static TValue ChangeType<TValue>(string value)
{
return ChangeType<TValue>(value, CultureInfo.CurrentCulture);
}
}
}
Also read https://dotnetcoder.com/create-json-localization-service-blazor-server/
Creating the DncOption component
In the Select folder, create a new file named DncOption.razor
and mark it as generic with a generic parameter named TValue
as in the following code.
@typeparam TValue
<option value="@Value" disabled="@Disabled" selected="@IsDefaultOption">
@if (ChildContent != null)
{
@ChildContent
}
else
{
@Value
}
</option>
@code {
[Parameter]
public TValue Value { get; set; }
[Parameter]
public RenderFragment ChildContent { get; set; }
[Parameter]
public bool IsDefaultOption { get; set; }
[Parameter]
public bool Disabled { get; set; }
}
The DncOption
component receives four parameters to be used in the markup.
The parameter ChildContent
of type RenderFragment
is used to inject embedded content into the component, or we display the the Value
if the ChildContent
is null.
The IsDefaultOption
and Disabled
parameters are used to set the default option and to disable an option.
Using the Blazor Select component in a Blazor Web App
To see our Blazor Select component in action, we will test it in a simple Blazor web app .
First, add a new Blazor Web App project template called Dnc.Select.WebApp
to the solution. I have selected .NET 8 (Long Term Support) as the target Framework.
Second reference the Dnc.Common.Razor
project to the Dnc.Select.WebApp
project, and update the _Imports.razor
as follows.
// Removed code for brevity
@using Dnc.Common.Razor.Select
Then include the bootstrap link in the App.razor
file, as shown below.
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.3/dist/css/bootstrap.min.css" rel="stylesheet">
Finally, add the following code to the Home.razor
file and run the application.
@page "/"
@using System.Text.Json
@using System.ComponentModel.DataAnnotations
@using System.Globalization
<PageTitle>Home</PageTitle>
<div class="container">
<p class=" alert alert-info my-3">@JsonSerializer.Serialize(MyEmployee);</p>
<EditForm Model="@MyEmployee" OnValidSubmit="HandleValidSubmit">
<label class="form-label my-3">Age</label>
<DncSelect @bind-Value="@MyEmployee.Age" TypeConverter="@((v) => int.Parse(v))">
<DncOption Value="default(int)" IsDefaultOption>Select Age</DncOption>
<DncOption Value="30" />
<DncOption Value="40" />
<DncOption Value="50" Disabled />
</DncSelect>
<label class="form-label my-3">Gender</label>
<DncSelect @bind-Value="@MyEmployee.Gender" TypeConverter="@(v=> Enum.Parse<Gender>(v))">
<DncOption Value="default(Gender)" IsDefaultOption="true">Select Gender</DncOption>
<DncOption Value="@Gender.Male" />
<DncOption Value="@Gender.Female" />
</DncSelect>
<label class="form-label my-3">Contract Expiration</label>
<DncSelect @bind-Value="@MyEmployee.ContractTill" CultureInfo="@(new CultureInfo("en-IE"))">
<DncOption Value="default(DateTime)" IsDefaultOption="true">Select Contract Expiration</DncOption>
<DncOption Value="@(new DateTime(2030, 7,7))">
@(new DateTime(2030, 7, 7).ToString("yyyy-mm-dd"))
</DncOption>
<DncOption Value="@(new DateTime(2035, 7,7))">
@(new DateTime(2035, 7, 7).ToString("yyyy-mm-dd"))
</DncOption>
</DncSelect>
<div class="form-group my-5">
<button class="btn btn-primary">Submit</button>
</div>
</EditForm>
</div>
@code{
public Employee MyEmployee {get; set;}
protected override void OnInitialized()
{
MyEmployee = new Employee
{
Name = "Net Coder",
};
}
public void HandleValidSubmit()
{
// insert it to the database
}
public class Employee {
public string Name { get; set; }
public int Age { get; set; }
public Gender Gender { get; set; }
public DateTime ContractTill { get; set; }
}
public enum Gender {
Male,
Female
}
}
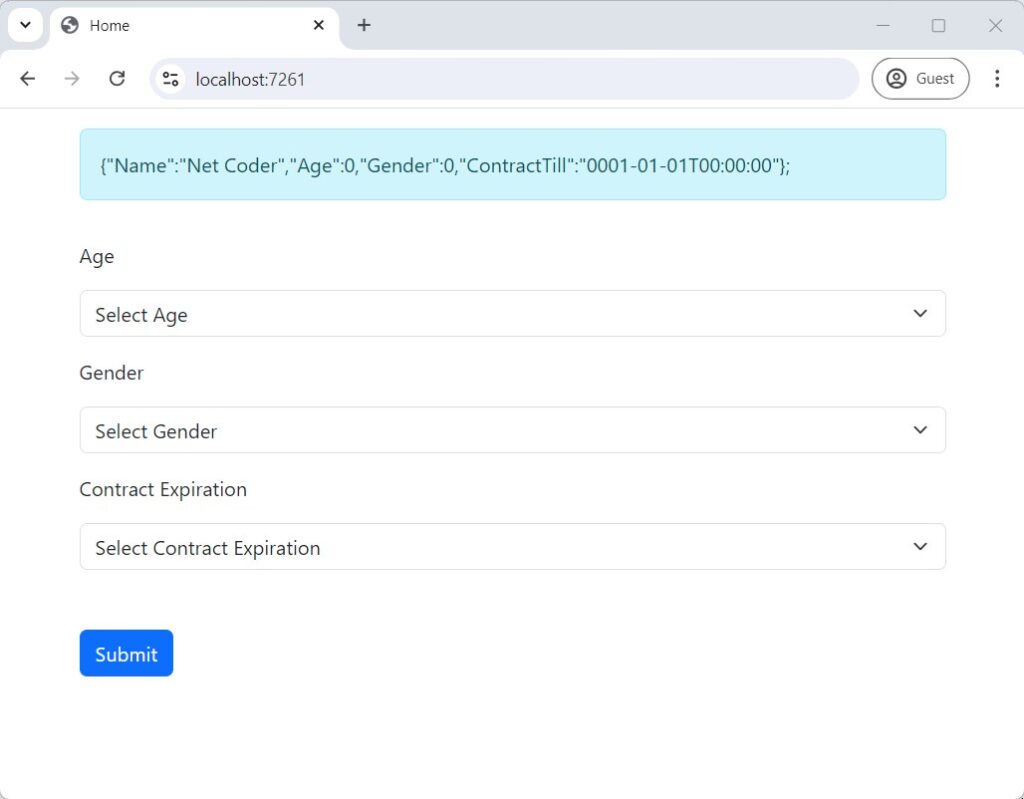
Conclusion
By creating a reusable components in Blazor, you can keep your code clean and maintainable. In this post, we created a Blazor Select component that you can use in your various projects. This component can be extended to meet your project requirements.
Also read https://dotnetcoder.com/creating-in-memory-cache-service/
Sample Code
You can find the complete sample code for this project on my GitHub repository.
Enjoy This Blog?
Discover more from Dot Net Coder
Subscribe to get the latest posts sent to your email.