Table of Contents
Client Credentials Flow in Entra ID Example
Take a look at the finished Client Credentials Flow in Entra ID Example implemented in .Net 8 and Entra ID ( Azure Ad).
What are Client Credentials Flow?
The Client Credentials flow is a flow that does not support user authentication. This type of flow is typically used for server-to-server interactions running in the background. It is also for calls that do not involve an end user. In simple words, the Client Credentials Flow is used when the client is a backend component. An example is an API calling another API. No interactive user is involved.
Client Credentials Flow Diagram
The following diagram shows Client Credentials Flow in Entra ID in detail.
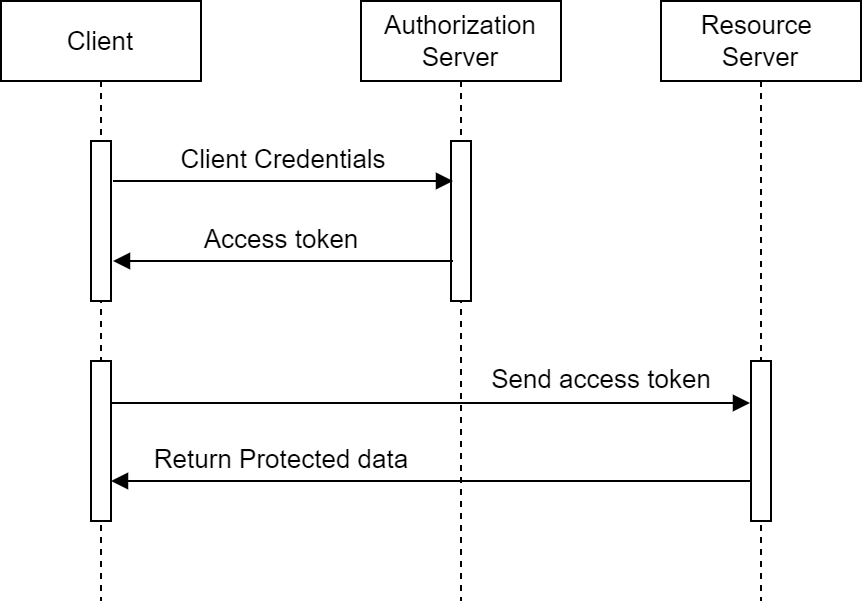
The client credentials flow in Entra ID consists of only a single request to the authorization server token. The client sends Client Id and Client Secret to the authorization server token to obtain an access token that we can send to a resource server (e.g. API) to return the protected data. The access tokens from the client credentials flow do not contain any user information.
When to use Client Credentials flow?
The client credentials flow in Entra ID is generally used in scenarios where no users are involved. The client acts as a backend component, e.g., an API calling an API or daemon apps that access an API. The access tokens from the Client Credentials Flow do not contain any user information. Therefore, you should not use the flow in scenarios where users are present.
Setting up the project
To implement the Client Credentials Flow in Entra ID in practice , open Visual Studio and create a blank solution named ClientCredentials
.
Then add a new ASP.NET Core Web API project template named Dnc.SecuredApi
and select .NET 8 (Long Term Support) as the target Framework. and then add a new console application named Dnc.ConsoleClient
to the ClientCredentials
as shown below.
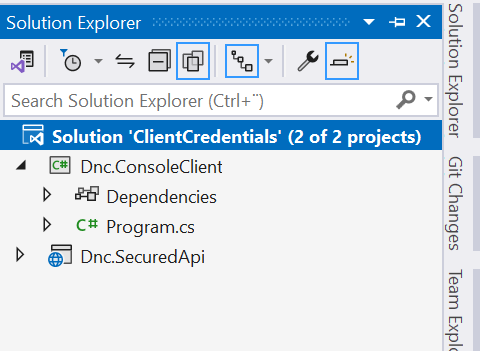
Registering the Web API in Microsoft Entra ID (Azure Ad)
You need An Azure account, subscription, go to the Azure Portal .
Go to Microsoft Entra ID in the left navigation pane. Click App Registrations and then click New Registration. Finally, enter the following information as shown below.
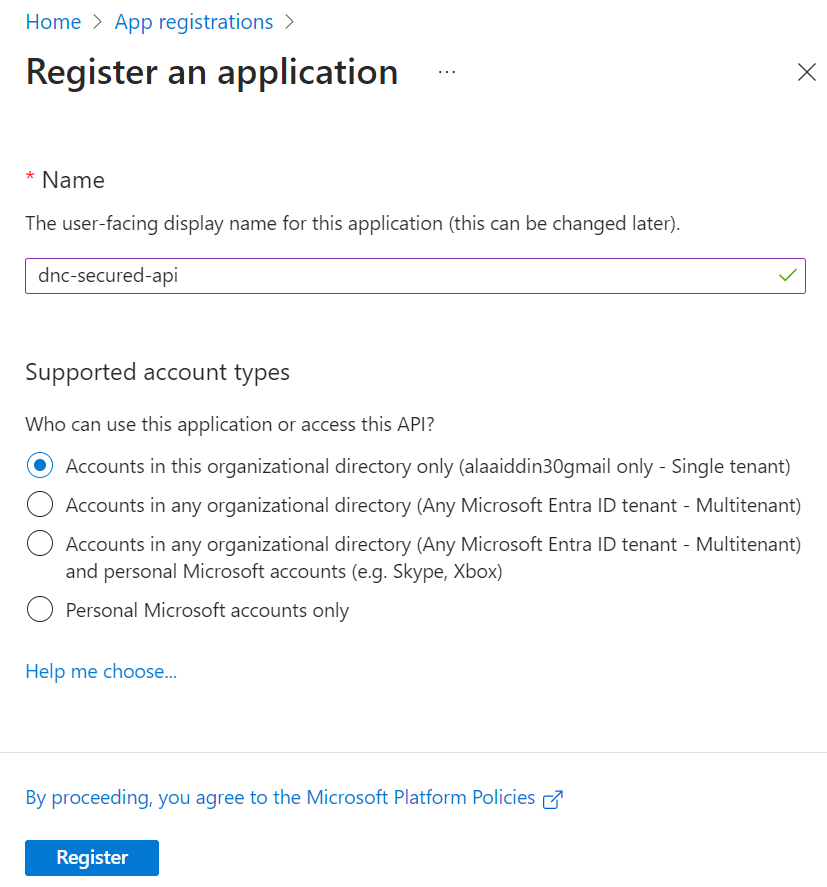
Click Register.
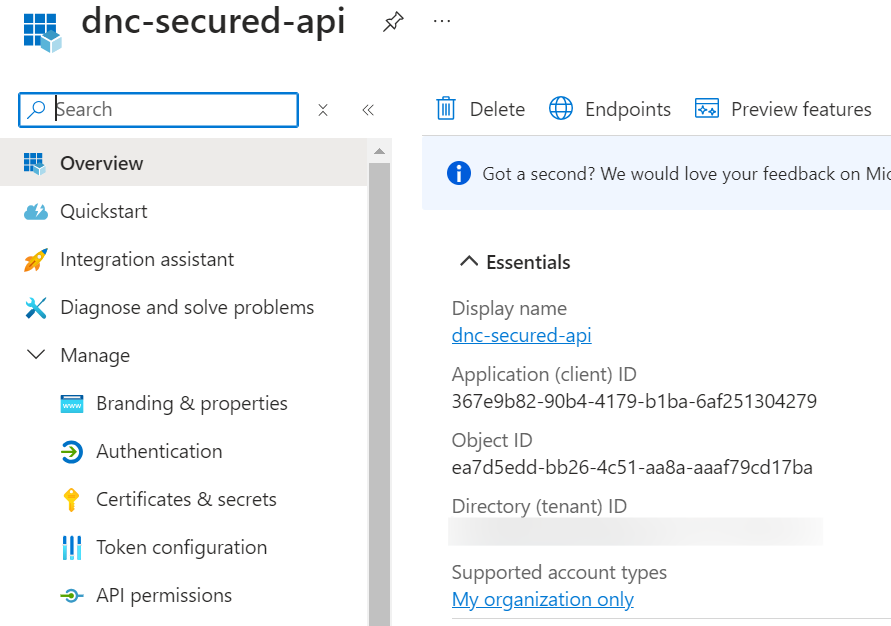
We now need to expose our API to make it consumable by setting an application permission. We must use application permissions, also known as app roles, that are granted by an admin or by the API’s owner because there is no user present in Client Credentials Flow in Entra ID scenario .
Go to “Expose an API”, set the Application ID URI (use something like api://{clientId}
) and click “Save” .
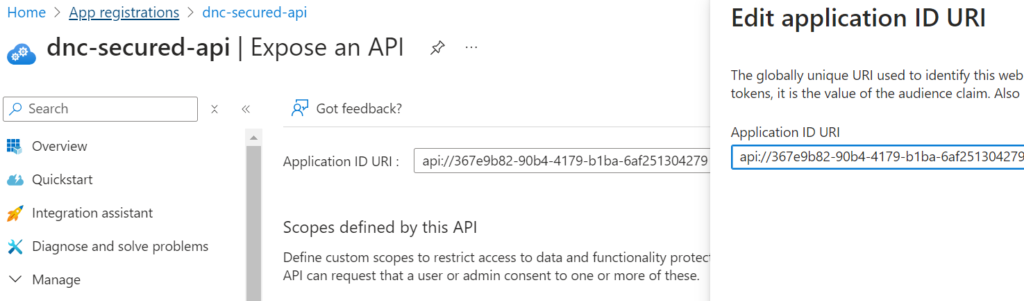
Under manage select App roles, and then select Create app role, enter the required information and click Apply as shown below.
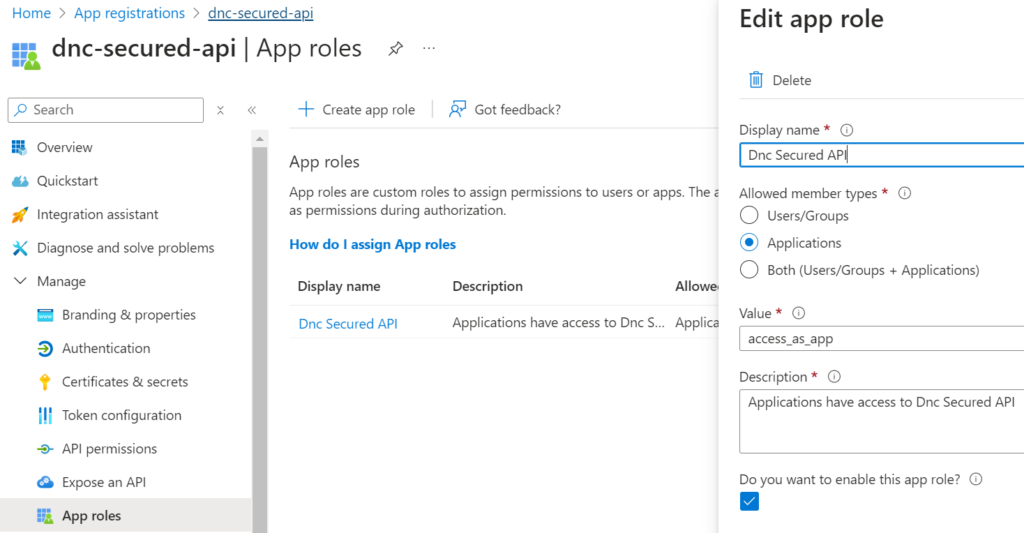
The next step is to protect our ASP.NET Core Web API 8 with Microsoft Entra ID.
Set up the ASP.NET Core 8 API
We need to protect the API our ASP.NET Core Web API 8 with Microsoft Entra ID.
Configure the Microsoft Entra ID credentials in the appsettings.Development.json
file as shown below.
{
"EntraId": {
"Instance": "https://login.microsoftonline.com",
"Domain": "{your-domain}",
"TenantId": "{your-tenant-id}",
"ClientId": "367e9b82-90b4-4179-b1ba-6af251304279",
"Audience": "api://367e9b82-90b4-4179-b1ba-6af251304279",
"Scopes": "access_as_app"
}
}
Avoid storing security information in your code. Use Azure Key Vault to securely manage the secret. Do not embed it directly in your code.
Second, add the required NuGet packages with the following commands.
dotnet add package Microsoft.Identity.Web
dotnet add package Microsoft.AspNetCore.Authentication.JwtBearer
Finally, update the Program.cs
file by adding the following code.
// Removed code for brevity
builder.Services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme)
.AddMicrosoftIdentityWebApi(bearerOptions =>
{
bearerOptions.TokenValidationParameters.ValidateAudience = true;
bearerOptions.Audience = builder.Configuration.GetValue<string>("EntraId:Audience");
bearerOptions.TokenValidationParameters = new TokenValidationParameters
{
ValidateIssuer = true,
ValidIssuer = $"https://login.microsoftonline.com/{builder.Configuration["EntraId:TenantId"]}/v2.0",
};
}, identityOptions =>
{
identityOptions.Instance = builder.Configuration.GetValue<string>("EntraId:Instance");
identityOptions.Domain = builder.Configuration.GetValue<string>("EntraId:Domain");
identityOptions.TenantId = builder.Configuration.GetValue<string>("EntraId:TenantId");
identityOptions.ClientId = builder.Configuration.GetValue<string>("EntraId:ClientId");
});
builder.Services.AddAuthorization(options =>
{
options.AddPolicy("AccessAsApp", policy =>
policy.RequireRole("access_as_app"));
});
app.UseAuthentication();
app.UseAuthorization();
// Removed code for brevity
The code above is designed to separate the concerns of bearer token validation and Microsoft Identity configuration.
We have also created a policy that can be applied at the action or controller level. This policy ensures that the API caller, which is the console app in this case, includes the expected application permission. This permission must be in the access token.
builder.Services.AddAuthorization(options =>
{
options.AddPolicy("AccessAsApp", policy =>
policy.RequireRole("access_as_app"));
});
Update the IEnumerable<WeatherForecast> Get()
as shown below.
[Authorize(Policy = "AccessAsApp")]
public IEnumerable<WeatherForecast> Get()
{
// Removed code for brevity
}
While the code below can be used, I personally prefer having greater control over token validation.
builder.Services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme)
.AddMicrosoftIdentityWebApi(builder.Configuration.GetSection("AzureAd"));
Also read https://dotnetcoder.com/creating-a-reusable-blazor-modal-component/
Registering the Console App in Microsoft Entra ID
In Microsoft Entra ID, go to the left navigation pane. Click App Registrations, then click New Registration. Finally, enter the next information as shown below.
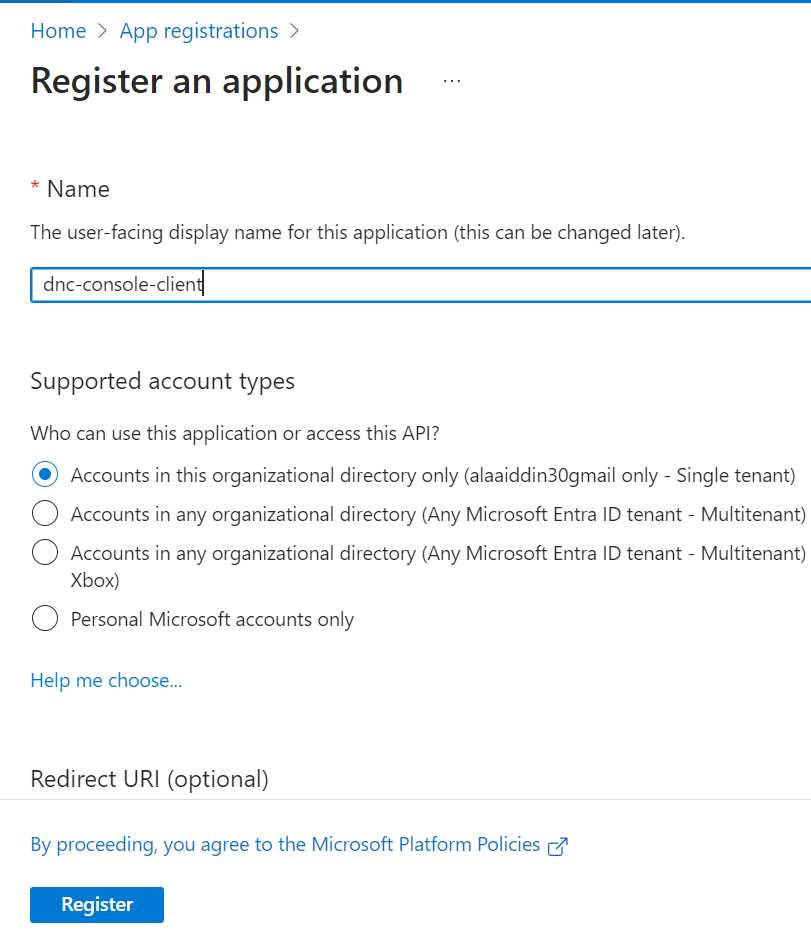
Click on “Register” and copy the required information for later use.
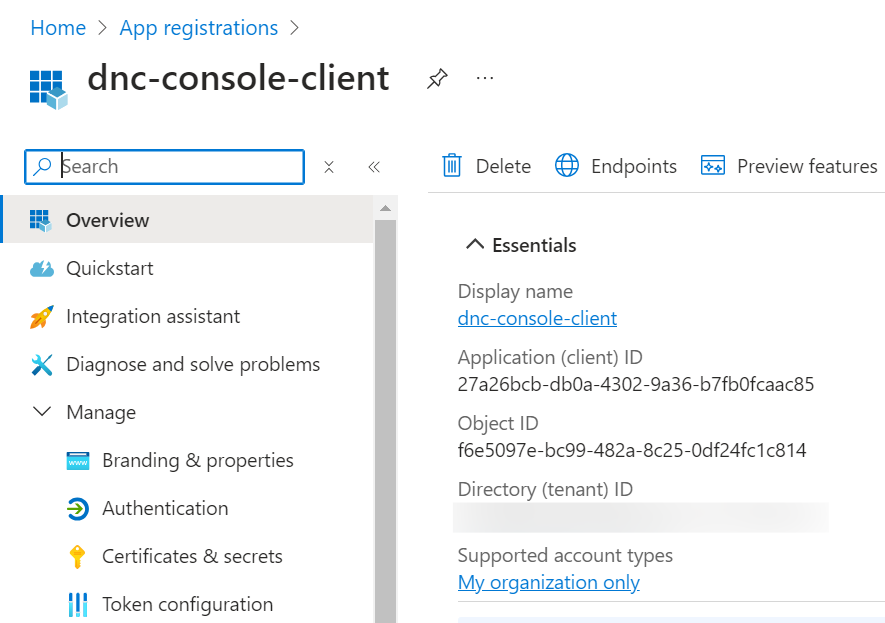
Second: Go to Certificate and secrets. Then go to New Client Secret and enter the required information. Click Add as shown below.
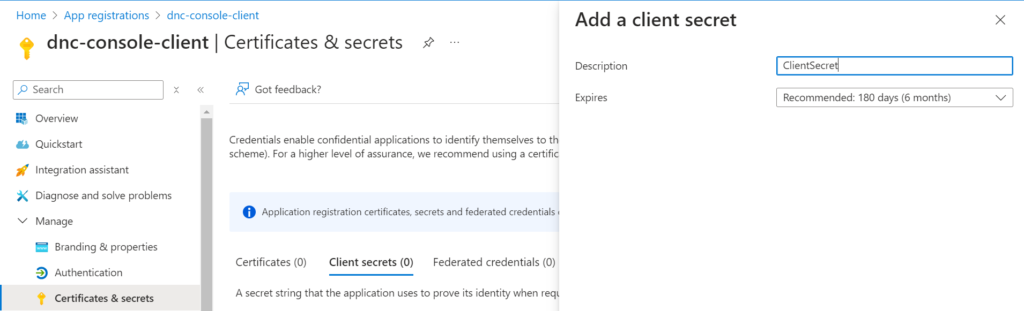
Copy the key and save it in a safe place. This key is no longer visible after you have closed the tile.
Finally, we need to assign app role to our console application in order to authenticate and make authorized API calls on its own, without requiring user interaction.
Go to API Permissions and then to Add a permission. Select the access_as_app
permission and click Add permissions as shown below.
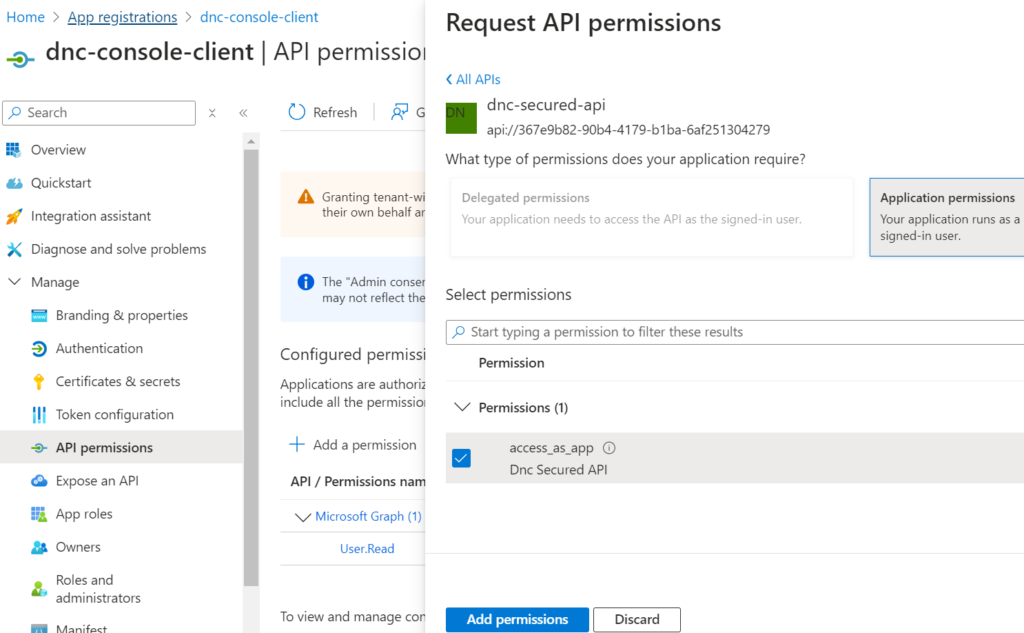
Because this is an application permission , an admin must grant consent to use the app role assigned to the console application by clicking on the Grant Admin Consent button as shown below.

Getting the access token and calling the API
The Azure configurations are ready for both console client and Web API is secure with Microsoft Management Identity. The next step is to configure our client to call the secure ASP.NET Core Web API 8 .
To better understand the Client Credentials Flow in Entra ID, you should know the basic form of HTTP messages. This knowledge can be helpful.
The authorization request uses a client_id
and client_secret
to authenticate on its own. It includes a parameter that tells the Authorization server what it should return. This parameter is grant_type=client_credentials
, as shown in the example below.
POST /{tenant}/oauth2/v2.0/token HTTP/1.1
Host: login.microsoftonline.com:443
Content-Type: application/x-www-form-urlencoded
client_id=27a26bcb-db0a-4302-9a36-b7fb0fcaac85
&scope=api%3A%2F%2367e9b82-90b4-4179-b1ba-6af251304279%2F.default
&client_secret=9X08Q~LZDyC6J96mg1fLLGzLjt0kjnCMK-Ffpcqn
&grant_type=client_credentials
Add the required NuGet package, with the following command.
dotnet add package Microsoft.Identity.Client
In the Dnc.ConsoleClient
project, update the Program.cs
as shown below.
namespace Dnc.ConsoleClient
{
internal class Program
{
private static readonly string clientSecret = "{your-client-secret}";
private static readonly string clientId = "{your-client-id}";
private static readonly string tenantId = "{your-tenant-id}";
private static readonly string[] scopes = ["api://{your-api-client-id}/.default"];
static async Task Main(string[] args)
{
string accessToken = await GetAccessTokenUsingHttpClient();
Console.WriteLine("Press Enter to Acquire Access Token using HttpClient (Credentials Flow)");
Console.ReadLine();
Console.WriteLine($"************Access Token Using HttpClient***************");
Console.WriteLine($"{accessToken}\n");
var data = await GetWeatherForecast(accessToken);
Console.WriteLine("Press Enter to call the secure web api");
Console.ReadLine();
Console.WriteLine($"************Response from the Secured API***************");
Console.WriteLine($"{data}\n");
string accessTokenUsingMSAL = await GetAccessTokenUsingMSAL();
Console.ForegroundColor = ConsoleColor.Green;
Console.WriteLine("Press Enter to Acquire Access Token using MSAL.NET (Credentials Flow)");
Console.ResetColor();
Console.ReadLine();
Console.ForegroundColor = ConsoleColor.Red;
Console.WriteLine($"************Access Token using MSAL.NET***************");
Console.ResetColor();
Console.WriteLine($"{accessTokenUsingMSAL}\n");
var data2 = await GetWeatherForecast(accessToken);
Console.ForegroundColor = ConsoleColor.Green;
Console.WriteLine("Press Enter to call the secure web api");
Console.ResetColor();
Console.ReadLine();
Console.ForegroundColor = ConsoleColor.Red;
Console.WriteLine($"************Response from the Secured API***************");
Console.ResetColor();
Console.WriteLine($"{data2}");
}
//Using HttpClient to acquire token using the client credentials flow.
private static async Task<string> GetAccessTokenUsingHttpClient()
{
using var client = new HttpClient();
var tokenEndpoint = $"https://login.microsoftonline.com/{tenantId}/oauth2/v2.0/token";
var request = new HttpRequestMessage(HttpMethod.Post, tokenEndpoint);
var parameters = new Dictionary<string, string>
{
{"client_id", clientId },
{"client_secret", clientSecret},
{"scope", scopes[0]},
{"grant_type", "client_credentials"}
};
var content = new FormUrlEncodedContent(parameters);
request.Content = content;
var response = await client.SendAsync(request);
if (!response.IsSuccessStatusCode)
throw new HttpRequestException($"Request failed with status code {response.StatusCode}");
using var doc = JsonDocument.Parse(await response.Content.ReadAsStringAsync());
return doc.RootElement.TryGetProperty("access_token", out var access_token) ?
access_token.GetString() : throw new InvalidOperationException("access token not found.");
}
//Using MSAL.NET to acquire token using the client credentials flow.
private static async Task<string> GetAccessTokenUsingMSAL()
{
IConfidentialClientApplication app = ConfidentialClientApplicationBuilder.Create(clientId)
.WithClientSecret(clientSecret)
.WithAuthority(new Uri($"https://login.microsoftonline.com/{tenantId}"))
.Build();
AuthenticationResult result = await app.AcquireTokenForClient(scopes).ExecuteAsync();
return result.AccessToken;
}
private static async Task<string> GetWeatherForecast(string accessToken)
{
using var httpClient = new HttpClient { BaseAddress = new Uri("https://localhost:7157/") };
httpClient.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", accessToken);
return await httpClient.GetStringAsync("WeatherForecast");
}
}
}
In the code above, we have created two methods for retrieving the access token. The first method, GetAccessTokenUsingHttpClient
, utilizes the HttpClient
to send a POST
request to the authorization server’s token endpoint. This method includes the necessary parameters and returns the access token.
The second method, GetAccessTokenUsingMSAL
, utilizes MSAL.NET to acquire the token using the client credentials flow in Entra ID (Azure Ad).
Once we have obtained the access token, we can proceed to call our secure API. We do this by inserting the token into the Authorization header. This is demonstrated in the following method.
private static async Task<string> GetWeatherForecast(string accessToken)
{
using var httpClient = new HttpClient { BaseAddress = new Uri("https://localhost:7157/") };
httpClient.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", accessToken);
return await httpClient.GetStringAsync("WeatherForecast");
}
We need to run multiple projects at the same time in our Visual Studio 2022. To do this, select Properties. Then choose Multiple Startup Projects in the solution. Set the action for both projects to Start
. Finally, press F5 to launch.
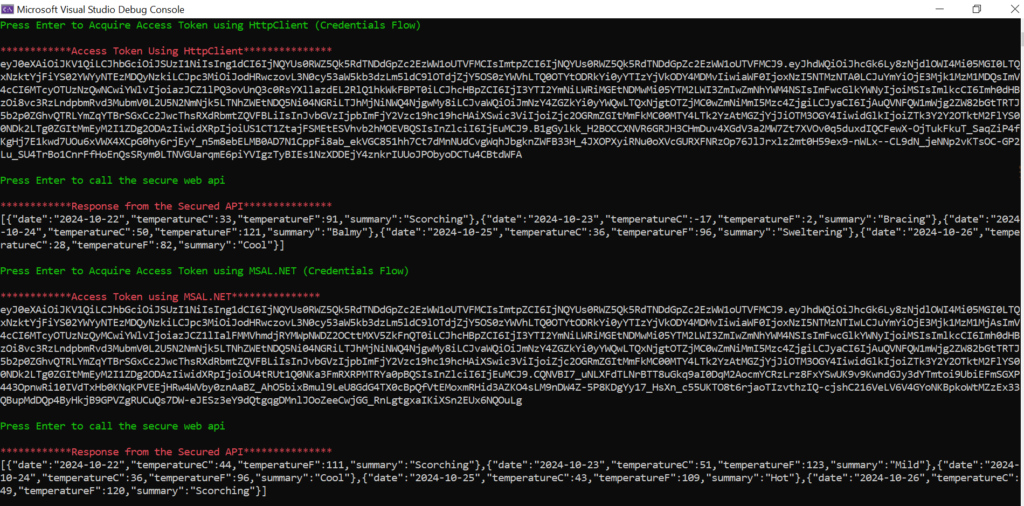
Conclusion
The Client Credentials Flow in Entra ID (Azure Ad) is an authentication mechanism that does not require user interaction. It is suitable for background services such as server-to-server and system integrations. The client credentials flow in Entra ID (Azure Ad) consists of only a single request to the authorization server token. The client sends Client Id and Client Secret to the authorization server token to obtain an access token that we can send to a resource server.
Also read https://dotnetcoder.com/creating-a-reusable-blazor-modal-component/
Sample code
You can find the full example code for this project on my GitHub repository
Enjoy This Blog?
Discover more from Dot Net Coder
Subscribe to get the latest posts sent to your email.