Table of Contents
What is HttpClient in .NET Core?
HttpClient is a class that sends HTTP requests (like GET, POST, PUT, and DELETE) and receives responses. It interacts with a resource identified by a URI.
In this post we will create a reusable HttpClient service in ASP.NET Core that can call secured ASP.NET Core Web APIs with Microsoft Entra ID (formerly Azure AD) . The reusable HttpClient service in ASP.NET Core handles different OAuth 2.0 flows depending on configuration.
HttpClient service in ASP.NET Core With Entra ID Example
Take a look at the finished HttpClient service in ASP.NET Core With Entra ID Example implemented in .Net 8 and Entra ID ( Azure Ad).
Setting up the project
Our project consists of two services that combined to implement the reusable HttpClient service with Entra ID.
The first service is EntraIdAuthenticationService
. It isolates the Azure Entra ID (Azure Ad) authentication logic.
To implement HttpClient
service in ASP.NET Core With Entra ID in practice, open Visual Studio. Create a blank solution named RestApiClient
. Add a new Class library named Dnc.Services.RestClient
to the solution. Select .NET 8 (Long Term Support) as the target Framework.
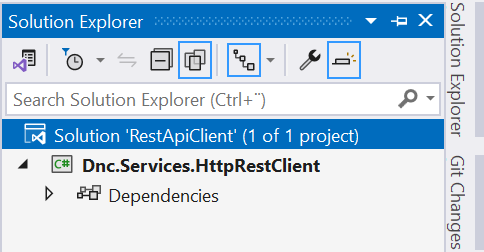
Create a new directory in the Dnc.Services.RestClient
project named EntraId
. Then add a new interface file named IEntraIdAuthenticationService.cs
as shown below.
namespace Dnc.Services.RestClient.EntraId
{
public interface IEntraIdAuthenticationService
{
// Web App calling API
Task<string> GetAccessTokenForUserAsync(IEnumerable<string> scopes);
// Client credentials flow
Task<string> GetAccessTokenForAppAsync(string scope);
// OBO flow
Task<string> AcquireAccessTokenOnBehalfOf(IEnumerable<string> scopes, string assertion);
}
}
The EntraIdAuthenticationService
service handles authentication based on whether it’s on behalf of the user or on behalf of the app.
Then add a new class file named EntraIdAuthenticationService.cs
, that implements the interface as shown below.
namespace Dnc.Services.RestClient.EntraId
{
public class EntraIdAuthenticationService : IEntraIdAuthenticationService
{
private readonly IConfidentialClientApplication confidentialClientApplication;
private readonly ITokenAcquisition tokenAcquisition;
public EntraIdAuthenticationService(EntraIdOptions options, ITokenAcquisition tokenAcquisition)
{
this.tokenAcquisition = tokenAcquisition;
var builder = ConfidentialClientApplicationBuilder.CreateWithApplicationOptions(new ConfidentialClientApplicationOptions
{
ClientId = options.ClientId,
ClientSecret = options.ClientSecret,
TenantId = options.TenantId
});
confidentialClientApplication = builder.Build();
}
// Acquire token for interactive user
public async Task<string> GetAccessTokenForUserAsync(IEnumerable<string> scopes)
{
try
{
return await tokenAcquisition.GetAccessTokenForUserAsync(scopes);
}
catch (MsalException ex)
{
throw new InvalidOperationException(ex.Message);
}
}
// Credential flow
public async Task<string> GetAccessTokenForAppAsync(string scope)
{
return await tokenAcquisition.GetAccessTokenForAppAsync(scope);
}
// OBO flow
public async Task<string> AcquireAccessTokenOnBehalfOf(IEnumerable<string> scopes, string assertion)
{
var builder = confidentialClientApplication.AcquireTokenOnBehalfOf(scopes, new UserAssertion(assertion));
var authResult = await builder.ExecuteAsync();
var accessToken = authResult.AccessToken;
return accessToken;
}
}
}
We will not dive into details. we have explored the Client Credentials Flow and On Behalf Of Flow in details in our earlier articles.
Finally we will create an extension method so we can set up our service easily in our Asp.Net Core Applications. To do that create a new directory in the Dnc.Services.RestClient
project named Options
. Then add a new class file named EntraIdOptions.cs
as shown below.
namespace Dnc.Services.RestClient.Options
{
public class EntraIdOptions
{
public string ClientId { get; set; }
public string ClientSecret { get; set; }
public string TenantId { get; set; }
}
}
Second : create a new directory in the Dnc.Services.RestClient
project named Extensions
. Then add a new class file named EntraIdExtensions.cs
as shown below.
namespace Dnc.Services.RestClient.Extensions
{
public static class EntraIdExtensions
{
public static IServiceCollection AddEntraIdAuthenticationService<TInterface, TImplementation>(
this IServiceCollection services,
Action<EntraIdOptions> configureOptions)
where TImplementation : TInterface, IEntraIdAuthenticationService
where TInterface : class
{
services.AddScoped<TInterface>(provider =>
{
var options = new EntraIdOptions();
configureOptions?.Invoke(options);
return ActivatorUtilities.CreateInstance<TImplementation>(provider, options);
});
return services;
}
}
}
Creating the HttpClient service in ASP.NET Core Integrated with Entra ID
To implement the HttpClient service in ASP.NET Core Integrated with Entra ID, we need to wrap the HttpClient service. Then, we must get the access token based on the context used for authentication.
First add a new file class named HttpRestClientBase.cs
in the Dnc.Services.RestClient
project and add the following code.
namespace Dnc.Services.RestClient
{
public abstract class HttpRestClientBase
{
protected HttpClient HttpClient;
protected IHttpContextAccessor HttpContextAccessor;
protected IEntraIdAuthenticationService AzureAdAuthenticationService;
protected string UserScope;
protected string AppScope;
protected string Audience;
protected void ConfigureRestClient(Action<RestClientOptions> configureOptions)
{
var options = new RestClientOptions();
configureOptions(options);
HttpClient = options.HttpClient;
UserScope = options.UserScope;
AppScope = options.AppScope;
Audience = options.Audience;
HttpContextAccessor = options.ServiceProvider.GetRequiredService<IHttpContextAccessor>();
AzureAdAuthenticationService = options.ServiceProvider.GetRequiredService<IEntraIdAuthenticationService>();
}
public async Task<TResult> GetAsync<TResult>(string path) where TResult : class
{
var request = await CreateRequestMessage(HttpMethod.Get, path);
var response = await HttpClient.SendAsync(request);
var content = await response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
var options = new JsonSerializerOptions
{
PropertyNamingPolicy = JsonNamingPolicy.CamelCase,
PropertyNameCaseInsensitive = true,
DefaultIgnoreCondition = JsonIgnoreCondition.WhenWritingNull,
};
return JsonSerializer.Deserialize<TResult>(content, options);
}
else
{
throw new HttpRequestException($"Request failed with status {response.StatusCode}: {content}");
}
}
public async Task<T> PostAsync<T>(string uri, object payload = null,
Dictionary<string, string> headers = null)
{
var request = await CreateRequestMessage(HttpMethod.Post, uri);
request = HandleContent(request, payload, headers);
var response = await HttpClient.SendAsync(request);
var content = await response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
return JsonSerializer.Deserialize<T>(content);
}
else
{
throw new HttpRequestException($"Request failed with status {response.StatusCode}: {content}");
}
}
public async Task<T> PutAsync<T>(string uri, object payload = null,
Dictionary<string, string> headers = null)
{
var request = await CreateRequestMessage(HttpMethod.Put, uri);
request = HandleContent(request, payload, headers);
var response = await HttpClient.SendAsync(request);
var content = await response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
return JsonSerializer.Deserialize<T>(content);
}
else
{
throw new HttpRequestException($"Request failed with status {response.StatusCode}: {content}");
}
}
private async Task<HttpRequestMessage> CreateRequestMessage(HttpMethod method, string requestUri)
{
var request = new HttpRequestMessage(method, requestUri);
var accessToken = await AcquireAccessTokenBasedOnContext();
request.Headers.Authorization = new AuthenticationHeaderValue("Bearer", accessToken);
return request;
}
private static HttpRequestMessage HandleContent(HttpRequestMessage request, object payload = null,
Dictionary<string, string> headers = null)
{
// Handling different content types
if (payload is MultipartFormDataContent multipartData)
{
request.Content = multipartData;
}
else if (payload != null && payload is string || payload.GetType() == typeof(string))
{
var json = payload.GetType() == typeof(string) ? payload as string : JsonSerializer.Serialize(payload);
request.Content = new StringContent(json, Encoding.UTF8, "application/json");
}
else if (payload != null)
{
request.Content = new StringContent(payload.ToString(), Encoding.UTF8, "application/x-www-form-urlencoded");
}
if (headers != null)
{
foreach (var header in headers.Where(v => !string.IsNullOrWhiteSpace(v.Value)))
{
request.Headers.Remove(header.Key);
request.Headers.TryAddWithoutValidation(header.Key, header.Value);
}
}
return request;
}
private async Task<string> AcquireAccessTokenBasedOnContext()
{
var user = HttpContextAccessor.HttpContext?.User;
string accessToken;
if (user == null || !user.Identity.IsAuthenticated)
{
// No authenticated user, service-to-service scenario
accessToken = await AzureAdAuthenticationService.GetAccessTokenForAppAsync($"api://{Audience}/{AppScope}");
}
else
{
var inboundToken = GetInboundTokenFromRequest();
accessToken = string.IsNullOrEmpty(inboundToken)
? await AzureAdAuthenticationService.GetAccessTokenForUserAsync([$"api://{Audience}/{UserScope}"])
: await AzureAdAuthenticationService.AcquireAccessTokenOnBehalfOf([$"api://{Audience}/{UserScope}"], inboundToken);
}
return accessToken;
}
private string GetInboundTokenFromRequest()
{
var inboundToken = HttpContextAccessor.HttpContext?.Request?.Headers?.Authorization.FirstOrDefault();
if (inboundToken != null && inboundToken.StartsWith("Bearer "))
{
return inboundToken[7..];
}
return null;
}
}
}
HttpRestClientBase
receives IEntraIdAuthenticationService
and HttpClient
instances via dependency injection.
The AcquireAccessTokenBasedOnContext
method is responsible for acquiring the token based on the incoming request.
The ConfigureRestClient
method is responsible for configuring the HttpRestClientBase
, which is used in any service that inherits from the HttpRestClientBase
, as you will see in the HttpClient service in ASP.NET Core Integrated with Entra ID usage section.
The CreateRequestMessage
method is responsible for creating the outgoing request. It calls the AcquireAccessTokenBasedOnContext
method. Finally, it prepares the request by attaching the token using the Authorization
header.
The other methods are just wrapper around the HttpClient
methods.
Our HttpClient service in ASP.NET Core Integrated with Entra ID is ready. We need to create an extension method for easy configuration. Create a new class file named RestClientOptions.cs
in the Options directory and add the following code.
namespace Dnc.Services.RestClient.Options
{
public class RestClientOptions
{
public HttpClient HttpClient { get; set; }
public IServiceProvider ServiceProvider { get; set; }
public string BaseAddress { get; set; }
public string UserScope { get; set; }
public string AppScope { get; set; }
public string Audience { get; set; }
}
}
Second : add a new class file named RestClientExtensions.cs
in the Extensions directory and add the following code.
namespace Dnc.Services.RestClient.Extensions
{
public static class RestClientExtensions
{
public static IServiceCollection AddRestClientService<TInterface, TImplementation>(
this IServiceCollection services,
Action<RestClientOptions> configureOptions)
where TImplementation : HttpRestClientBase, TInterface
where TInterface : class
{
services.AddScoped<TInterface>(provider =>
{
var options = new RestClientOptions();
configureOptions?.Invoke(options);
return ActivatorUtilities.CreateInstance<TImplementation>(provider, options);
});
return services;
}
}
}
Also read https://dotnetcoder.com/aspnet-core-web-api-best-practices-and-tips/
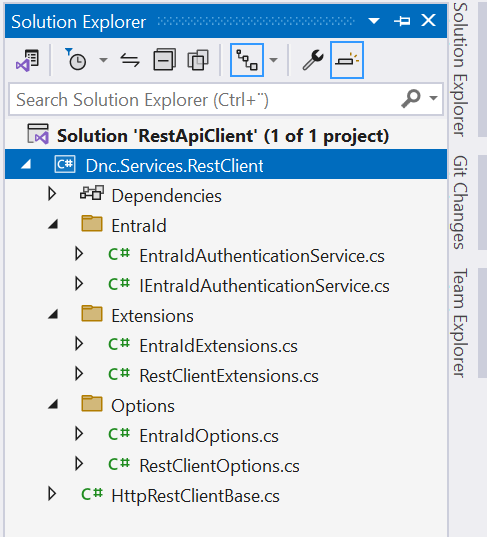
How to use the Reusable HttpClient service in ASP.NET Core Integrated with Entra ID
In this section we will discover how to use the reusable HttpClient service in ASP.NET Core Integrated with Entra ID by creating a Blazor Server Web App and two Asp.Net Core APIs as you see on below.
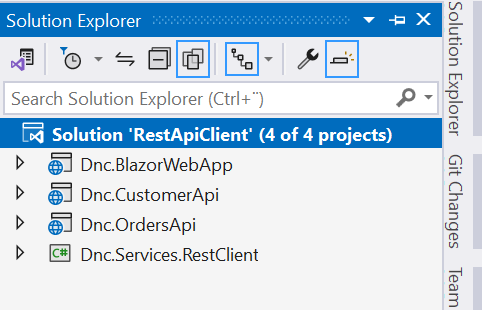
We have to register our APPs in Microsoft Entra ID and exposing the APIs with scopes.
I will not go through the App registrations and exposing the APIs ,
We have dived into details in our previous articles:
- Blazor Server App Authentication with Entra ID
- On Behalf Of Flow Entra ID
- Client Credentials Flow in Entra ID
We have three scenarios. The first scenario involves the BlazorWebApp
with a logged in user. The BlazorWebApp
calls the CustomerApi
to get a list of Customers. Then, the CustomerApi
calls the OrderApi
to get a list of Orders. (On Behalf Of flow).
In our first scenario the CustomerApi
exposes a scope called access_as_user
and the OrderAPi
exposes a scope named access_obo_user
.
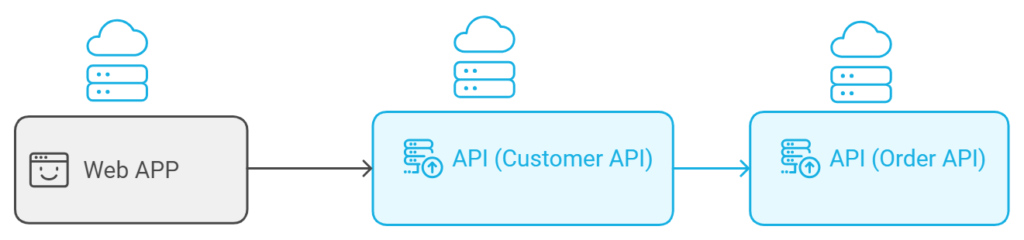
The second scenario involves the BlazorWebApp
with a logged in user. This app calls the CustomerApi
to get a list of Customers
, as an interactive user. In this scenario the CustomerApi
exposes a scope called access_as_user
.
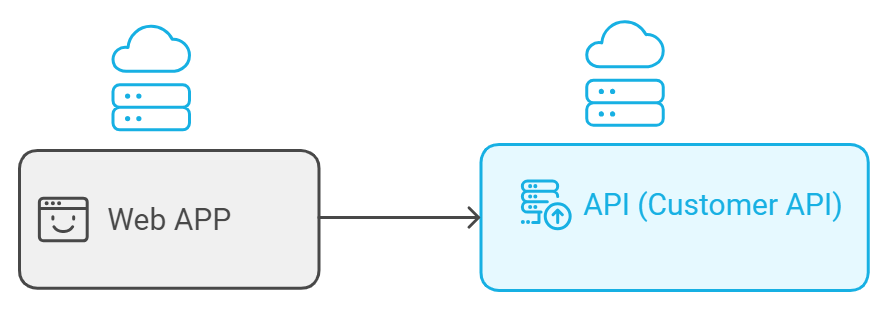
The third scenario involves the BlazorWebApp
pretending to be a service. It calls the CustomerApi
without user interaction. This is a service-to-service scenario using Client Credentials Flow. In this scenario the CustomerApi
is called with .default
scope.
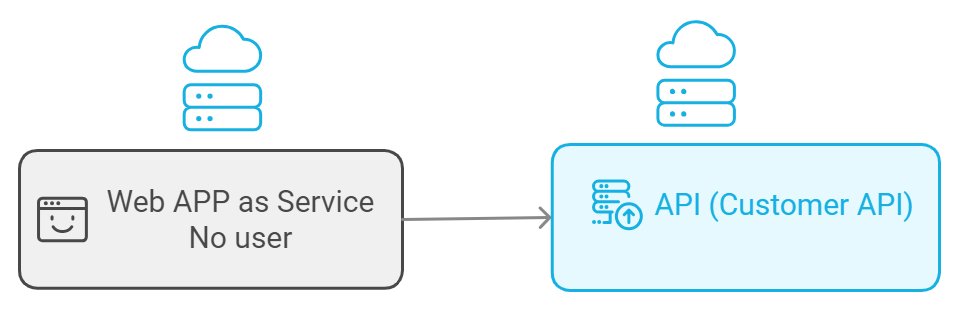
Configuring the Blazor Web App
Set up the Microsoft Entra ID credentials in the appsettings.Development.json
file as shown below.
{
"EntraId": {
"ClientId": "faa6297e-fde6-4966-9bc1-8b058426c2ce",
"ClientSecret": "Glg8Q~GrOA-DkjpfUDQykVl19iPGOkbPcgOf-bfg",
"Domain": "{your-domain}",
"TenantId": "{your-tenant-id}",
"ResponseType": "code",
"CallbackPath": "/signin-oidc",
"Instance": "https://login.microsoftonline.com/"
},
"CustomerApi": {
"CustomerApiClientId": "35afa630-29d5-4302-9273-2e8d48b49d3e",
"BaseAddress": "https://localhost:7151/api/",
"UserScope": "access_as_user",
"AppScope": ".default"
}
}
This information is needed to set up the HttpClient service HttpRestClientBase
implementation.
Avoid storing security information in your code. Use Azure Key Vault to securely manage the secret. Do not embed it directly in your code.
Then create a new directory in the Dnc.BlazorWebApp
project named Services
and add a new file named CustomerApiService.cs
as shown below
namespace Dnc.BlazorWebApp.Services
{
public interface ICustomerApiService
{
Task<IEnumerable<Customer>> GetCustomers();
Task<IEnumerable<Customer>> GetCustomersWithOrders();
}
public class CustomerApiService : HttpRestClientBase, ICustomerApiService
{
public CustomerApiService(RestClientOptions restOptions,
IHttpClientFactory httpClientFactory,
IServiceProvider provider)
{
var httpClient = httpClientFactory.CreateClient();
httpClient.BaseAddress = new Uri(restOptions.BaseAddress);
ConfigureRestClient(options =>
{
options.HttpClient = httpClient;
options.ServiceProvider = provider;
options.UserScope = restOptions.UserScope;
options.AppScope = restOptions.AppScope;
options.Audience = restOptions.Audience;
});
}
public async Task<IEnumerable<Customer>> GetCustomers()
{
return await GetAsync<IEnumerable<Customer>>("customers/all");
}
public async Task<IEnumerable<Customer>> GetCustomersWithOrders()
{
return await GetAsync<IEnumerable<Customer>>("customers/orders/all");
}
}
}
The CustomerApiService
implements HttpRestClientBase
and the ICustomerApiService
interfaces.
CustomerApiService
receives RestClientOptions
and IHttpClientFactory
instances via dependency injection, and the ConfigureRestClient
method configures the HttpClient service HttpRestClientBase
during the CustomerApiService
construction .
Finally, update the Program.cs
file as seen below.
// Blazor app authentication goes here
// Removed code for brevity
// Configure Services
builder.Services.AddHttpContextAccessor();
builder.Services.AddHttpClient();
builder.Services.AddEntraIdAuthenticationService<IEntraIdAuthenticationService, EntraIdAuthenticationService>(options =>
{
options.ClientId = builder.Configuration.GetValue<string>("EntraId:ClientId");
options.ClientSecret = builder.Configuration.GetValue<string>("EntraId:ClientSecret");
options.TenantId = builder.Configuration.GetValue<string>("EntraId:TenantId");
});
builder.Services.AddRestClientService<ICustomerApiService, CustomerApiService>(options =>
{
options.Audience = builder.Configuration.GetValue<string>("CustomerApi:CustomerApiClientId");
options.BaseAddress = builder.Configuration.GetValue<string>("CustomerApi:BaseAddress");
options.UserScope = builder.Configuration.GetValue<string>("CustomerApi:UserScope");
options.AppScope = builder.Configuration.GetValue<string>("CustomerApi:AppScope");
});
The Home.razor component after updating.
//The HTML is removed for brevity
@code {
private IEnumerable<Customer> Customers = [];
private bool loading = false;
private bool obo = false;
private async Task GetCustomers()
{
Customers = [];
loading = true;
Customers = await CustomerApiService.GetCustomers();
loading = false;
}
private async Task GetCustomersWithOrders()
{
obo = true;
Customers = [];
loading = true;
Customers = await CustomerApiService.GetCustomersWithOrders();
loading = false;
}
}
Configuring the CustomerApi
Set up the Microsoft Entra ID credentials in the appsettings.Development.json
file as shown below.
{
"AzureAd": {
"Instance": "https://login.microsoftonline.com",
"Domain": "{your-domain}",
"TenantId": "{your-tenant-id}",
"ClientId": "35afa630-29d5-4302-9273-2e8d48b49d3e",
"Audience": "api://35afa630-29d5-4302-9273-2e8d48b49d3e",
"Scopes": "access_as_user",
"ClientSecret": "AQk8Q~MWBMpbt-MDNpBKpVUfdCKdbMVDKu2~ibWa"
},
"OrderApi": {
"OrderApiClientId": "cca1c2f7-dda5-4de8-a616-d1ffce5af319",
"BaseAddress": "https://localhost:7194/api/",
"UserScope": "access_obo_user"
}
}
This information is needed to set up the HttpClient service HttpRestClientBase
implementation.
Avoid storing security information in your code. Use Azure Key Vault to securely manage the secret. Do not embed it directly in your code.
Then create a new directory in the Dnc.CustomerApi
project named Services
and add a new file named OrderApiService.cs
as shown below.
namespace Dnc.CustomerApi.Services
{
public interface IOrderApiService
{
Task<IEnumerable<Order>> GetOrders();
}
public class OrderApiService : HttpRestClientBase, IOrderApiService
{
public OrderApiService(RestClientOptions restOptions,
IHttpClientFactory httpClientFactory,
IServiceProvider provider)
{
var httpClient = httpClientFactory.CreateClient();
httpClient.BaseAddress = new Uri(restOptions.BaseAddress);
ConfigureRestClient(options =>
{
options.HttpClient = httpClient;
options.ServiceProvider = provider;
options.UserScope = restOptions.UserScope;
options.AppScope = restOptions.AppScope;
options.Audience = restOptions.Audience;
});
}
public async Task<IEnumerable<Order>> GetOrders()
{
return await GetAsync<IEnumerable<Order>>("orders/all");
}
}
}
In the same way , the ConfigureRestClient
method configures the HttpClient service HttpRestClientBase
during the OrderApiService
construction .
Finally, update the Program.cs
file as seen below.
builder.Services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme)
.AddMicrosoftIdentityWebApi(builder.Configuration.GetSection("AzureAd"))
.EnableTokenAcquisitionToCallDownstreamApi()
.AddInMemoryTokenCaches();
// Removed code for brevity
// Configure RestClient service
builder.Services.AddHttpContextAccessor();
builder.Services.AddHttpClient();
builder.Services.AddEntraIdAuthenticationService<IEntraIdAuthenticationService, EntraIdAuthenticationService>(options =>
{
options.ClientId = builder.Configuration.GetValue<string>("AzureAd:ClientId");
options.ClientSecret = builder.Configuration.GetValue<string>("AzureAd:ClientSecret");
options.TenantId = builder.Configuration.GetValue<string>("AzureAd:TenantId");
});
builder.Services.AddRestClientService<IOrderApiService, OrderApiService>(options =>
{
options.Audience = builder.Configuration.GetValue<string>("OrderApi:OrderApiClientId");
options.UserScope = builder.Configuration.GetValue<string>("OrderApi:UserScope");
options.BaseAddress = builder.Configuration.GetValue<string>("OrderApi:BaseAddress");
});
Create a new controller in the Dnc.CustomerApi
project named CustomerController.cs
, and add the following code.
namespace Dnc.CustomerApi.Controllers
{
[ApiController]
[Authorize]
[Route("api/customers")]
public class CustomerController : ControllerBase
{
private readonly IOrderApiService orderApiService;
public CustomerController(IOrderApiService orderApiService)
{
this.orderApiService = orderApiService;
}
[HttpGet("all")]
public IEnumerable<Customer> GetCustomers()
{
return
[ new Customer { Id = 1, FirstName = "John", LastName = "Doe", Email = "john.doe@example.com" },
new Customer { Id = 2, FirstName = "Jane", LastName = "Smith", Email = "jane.smith@example.com" },
new Customer { Id = 3, FirstName = "Emily", LastName = "Joe", Email = "emily.Joe@example.com" }
];
}
[HttpGet("orders/all")]
[RequiredScope(RequiredScopesConfigurationKey = "AzureAd:scopes")]
public async Task<IEnumerable<Customer>?> GetCustomersWithOrders()
{
var orders = await orderApiService.GetOrders();
if (orders.Any())
{
return
[ new Customer { Id = 1, FirstName = "John", LastName = "Doe", Email = "john.doe@example.com", Orders= orders.Where(v=>v.CustomerId == 1) },
new Customer { Id = 2, FirstName = "Jane", LastName = "Smith", Email = "jane.smith@example.com", Orders= orders.Where(v=>v.CustomerId == 2)},
new Customer { Id = 3, FirstName = "Emily", LastName = "Joe", Email = "emily.Joe@example.com", Orders= orders.Where(v=>v.CustomerId == 3) }
];
}
return null;
}
}
}
Configuring the OrderApi
Update the appsettings.Development.json
with the following code.
"AzureAd": {
"Instance": "https://login.microsoftonline.com",
"Domain": "{your-domain}",
"TenantId": "{your-tenant-id}",
"ClientId": "cca1c2f7-dda5-4de8-a616-d1ffce5af319",
"Audience": "api://cca1c2f7-dda5-4de8-a616-d1ffce5af319",
"Scopes": "access_obo_user"
}
Then update the Program.cs
file as seen below.
builder.Services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme)
.AddMicrosoftIdentityWebApi(builder.Configuration.GetSection("AzureAd"));
Create a new controller in the Dnc.OrderApi
project named OrderController.cs
, and add the following code.
Also read https://dotnetcoder.com/on-behalf-of-flow-entra-id/
namespace Dnc.OrdersApi.Controllers
{
[ApiController]
[RequiredScope(RequiredScopesConfigurationKey = "AzureAd:scopes")]
[Authorize]
[Route("api/orders")]
public class OrderController : ControllerBase
{
[HttpGet("all")]
public IEnumerable<Order> GetOrders()
{
return
[
new Order { OrderId = 102, CustomerId = 1, TotalAmount = 75.00m },
new Order { OrderId = 101, CustomerId = 1, TotalAmount = 250.00m },
new Order { OrderId = 201, CustomerId = 2, TotalAmount = 200.00m },
new Order { OrderId = 102, CustomerId = 2, TotalAmount = 150.50m },
new Order { OrderId = 103, CustomerId = 3, TotalAmount = 325.75m },
new Order { OrderId = 302, CustomerId = 3, TotalAmount = 200.00m }
];
}
}
}
HttpClient service in ASP.NET Core Integrated with Entra ID in action.
We need to run multiple projects at the same time in our Visual Studio 2022. To do this, select Properties. Then choose Multiple Startup Projects in the solution. Set the action for the BlazorWebApp
, CustomerApi
and OrderApi
projects to Start
. Finally, press F5 to launch.
HttpClient service in ASP.NET Core Integrated with Entra ID in action (Client Credentials flow scenario).
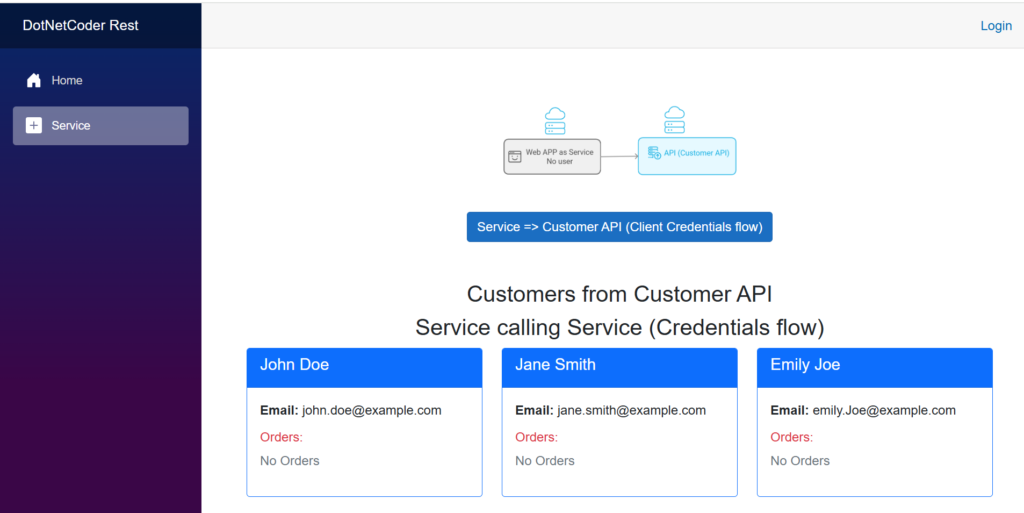
HttpClient service in ASP.NET Core Integrated with Entra ID in action (Web App calls API scenario).
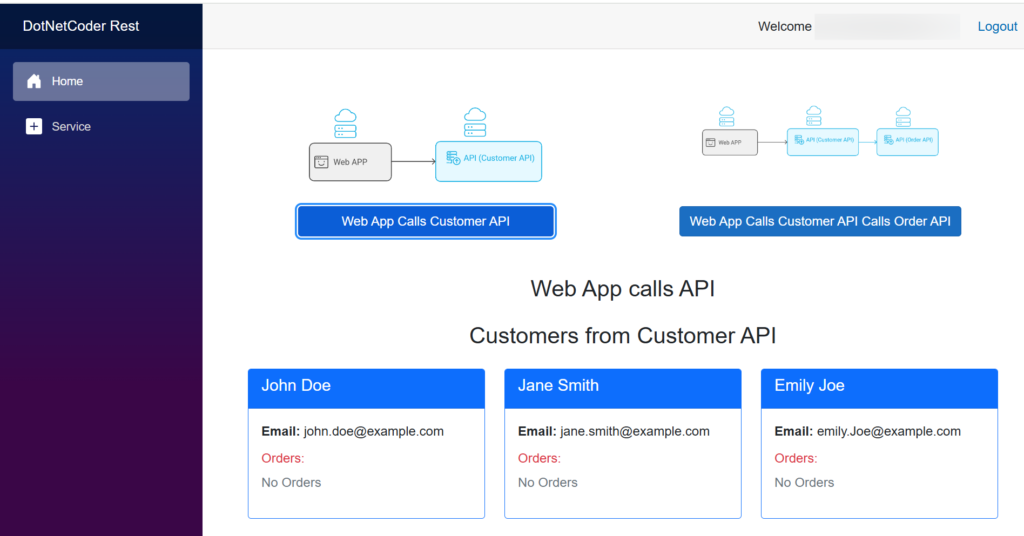
HttpClient service in ASP.NET Core Integrated with Entra ID in action (OBO flow scenario).
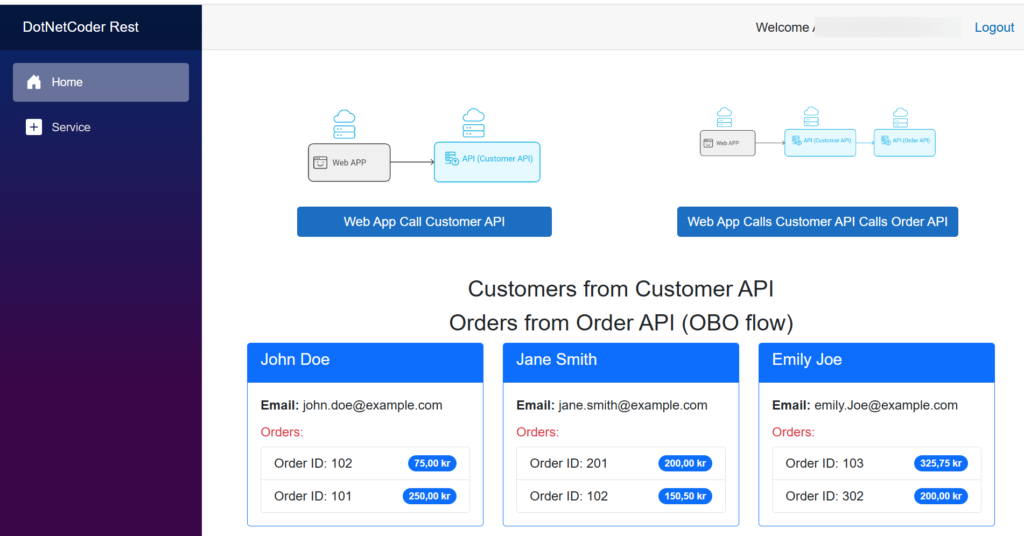
Conclusion
In this post, we created a Reusable HttpClient service in ASP.NET Core Integrated with Entra ID. You can user it in various projects and lets you easily make secure API calls from ASP.NET Core to APIs protected by Microsoft Entra ID and provides flexibility and security for enterprise applications.
Sample code
You can find the entire example code for Reusable HttpClient service in ASP.NET Core Integrated with Entra ID project on my GitHub repository
Enjoy This Blog?
Discover more from Dot Net Coder
Subscribe to get the latest posts sent to your email.