Table of Contents
Introduction
A Blazor confirm dialog component is a reusable UI element that asks users to confirm or accept an action. It is typically used for critical actions like deleting data. When triggered, a modal dialog pops up containing a message to ask the user that they are about to take a dangerous action that requires confirmation before processing.
Creating a Confirm Solution and adding a Razor class library project
Open Visual Studio and create a blank solution named Confirm
. Add a new Razor Class library named Dnc.Common.Razor
to the solution. Select .NET 8 (Long Term Support) as the target Framework.
Creating the DncConfirm component
First, create a new folder in the Dnc.Common.Razor
project named Confirm. Then, create a new class file derived from the ComponentBase
class called DncConfirmComponent.cs
.
namespace Dnc.Common.Razor.Confirm
{
public class DncConfirmComponent: ComponentBase
{
[Parameter] public RenderFragment HeaderTemplate { get; set; }
[Parameter] public RenderFragment BodyTemplate { get; set; }
[Parameter] public RenderFragment OkTemplate { get; set; }
[Parameter] public RenderFragment CancelTemplate { get; set; }
[Parameter] public bool Scrollable { get; set; }
[Parameter] public EventCallback<bool> OnOk { get; set; }
protected bool Visible { get; set; }
public void Display()
{
Visible = true;
StateHasChanged();
}
protected void Cancel()
{
Visible = false;
}
protected async Task Ok()
{
Visible = false;
await OnOk.InvokeAsync(true);
}
}
}
We will go over the code and provide some explanation.
[Parameter] public RenderFragment HeaderTemplate { get; set; }
[Parameter] public RenderFragment BodyTemplate { get; set; }
[Parameter] public RenderFragment OkTemplate { get; set; }
[Parameter] public RenderFragment CancelTemplate { get; set; }
[Parameter] public bool Scrollable { get; set; }
We defined four RenderFragment
parameter properties in the DncConfirmComponent
that allow us to inject the Blazor confirm dialog’ header, body and buttons content into the component.
The dialog can be made scrollable by setting the Scrollable
parameter.
[Parameter] public EventCallback<bool> OnOk { get; set; }
protected bool Visible { get; set; }
public void Display()
{
Visible = true;
StateHasChanged();
}
protected void Cancel()
{
Visible = false;
}
protected async Task Ok()
{
Visible = false;
await OnOk.InvokeAsync(true);
}
The EventCallback OnOk
is used to communicate between the DncConfirmComponent
and the parent component. In our case, it will handle the click event raised by the Blazor dialog component.
The Display
method sets the Visible
property to true
, causing the dialog to pop up and notifying the component to be re-rendered.
The markup of the Confirm component includes some HTML and Bootstrap CSS styles, and it is self-explanatory.
In the Confirm folder, create a new file called DncConfirm.razor
, which inherits from the DncConfirmComponent
class, and enter the following markup.
@inherits DncConfirmComponent
@if (Visible)
{
<div class="modal fade show confirm-background" id="DncConfirm" style="display: block;" aria-modal="true" role="dialog">
<div class="modal-dialog @(Scrollable ? " modal-dialog-scrollable":"")">
<div class="modal-content">
<div class="modal-header">
@if (HeaderTemplate == null)
{
<h5 class="modal-title" id="exampleModalLabel">Confirm title</h5>
}
else
{
<h5>@HeaderTemplate</h5>
}
<button type="button" class="btn-close" data-bs-dismiss="modal" aria-label="Close" @onclick="@Cancel"></button>
</div>
<div class="modal-body">
@if (BodyTemplate == null)
{
<p>You're reading the default text in a confirmation body!</p>
}
else
{
<p>@BodyTemplate</p>
}
</div>
<div class="modal-footer">
<button type="button" class="btn btn-secondary confirm-button" data-bs-dismiss="modal" @onclick="@Cancel">
@if (CancelTemplate == null)
{
<span>Cancel</span>
}
else
{
@CancelTemplate
}
</button>
<button type="button" class="btn btn-primary confirm-button" @onclick=@Ok>
@if (OkTemplate == null)
{
<span>Ok</span>
}
else
{
@OkTemplate
}
</button>
</div>
</div>
</div>
</div>
}
Finally, create a new CSS file called DncConfirm.razor.css
, and enter the following code.
.modal-dialog {
margin-top: 7rem;
}
.confirm-background {
background-color: rgba(0, 0, 0, 0.4);
backdrop-filter: blur(15px);
}
.confirm-button {
min-width: 6rem;
}
Also read https://dotnetcoder.com/entity-framework-code-first-approach-in-net-8/
Using the Blazor Confirm Dialog in a Blazor Web App
In this section we will learn how to use our custom Blazor Confirm Dialog in the Blazor application.
First, add a new Blazor Web App project template named Dnc.Confirm.WebApp
to the solution. Select .NET 8 (Long Term Support) as the target Framework and set it as Startup project.
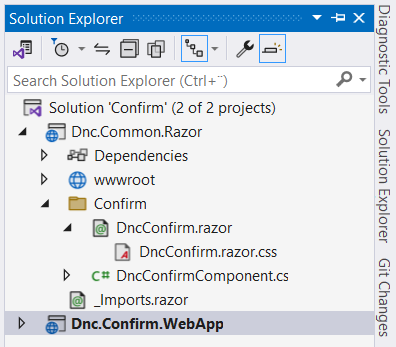
Second reference the Dnc.Common.Razor
project to the Dnc.Confirm.WebApp
project, and edit the _Imports.razor
as follows.
// Removed code for brevity
@using Dnc.Common.Razor.Confirm
Then include the bootstrap link in the App.razor
file, as shown below.
<head>
// Removed code for brevity
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.2/dist/css/bootstrap.min.css">
<HeadOutlet @rendermode="InteractiveServer" />
</head>
Finally, insert the following code into the Home.razor
and start the application.
@page "/"
<PageTitle>Home</PageTitle>
<style>
body
{
background-color:#eee;
}
</style>
<div class="container">
<div class="row">
<div class="col">
<h3>Accounts</h3>
<table class="table my-6" style="background-color:#fff;">
<thead><tr><th>Id</th><th>First name</th><th>Last name</th><th>Status</th><th>Expire date</th><th>#</th><th>#</th></tr></thead>
<tbody>
@foreach (var account in Accounts)
{
<tr>
<td>@account.Id</td>
<td>@account.FirstName</td>
<td>@account.LastName</td>
<td>@(account.Blocked ? "Blocked" : "Active")</td>
<td>@account.ExpireDate.ToShortDateString()</td>
<td><button class="btn btn-warning" @onclick="()=>ShowBlockAccount(account.Id)">Block account</button></td>
<td><button class="btn btn-danger" @onclick="()=>ShowDeleteAccount(account.Id)">Delete account</button></td>
</tr>
}
</tbody>
</table>
</div>
</div>
</div>
<DncConfirm @ref="ConfirmDeleteAccount" OnOk="()=>DeleteAccount()">
<HeaderTemplate>
Delete an account!
</HeaderTemplate>
<BodyTemplate>
Are you sure you want to delete this account ?
</BodyTemplate>
<OkTemplate>
Delete
</OkTemplate>
</DncConfirm>
<DncConfirm @ref="ConfirmBlockAccount" Scrollable="true" OnOk="()=>BlockAccount()">
<HeaderTemplate>
Block an account!
</HeaderTemplate>
<BodyTemplate>
Are you sure you want to Block this account ?
</BodyTemplate>
<OkTemplate>
Block
</OkTemplate>
</DncConfirm>
@code{
public DncConfirm ConfirmDeleteAccount { get; set; }
public DncConfirm ConfirmBlockAccount { get; set; }
private int AccountId { get; set; }
protected void ShowDeleteAccount(int id)
{
AccountId = id;
ConfirmDeleteAccount.Display();
}
protected void ShowBlockAccount(int id)
{
AccountId = id;
ConfirmBlockAccount.Display();
}
protected void DeleteAccount()
{
var account = Accounts.Where(v => v.Id == AccountId).FirstOrDefault();
if(account != null)
{
Accounts.Remove(account);
}
}
protected void BlockAccount()
{
var account = Accounts.Where(v => v.Id == AccountId).FirstOrDefault();
if (account != null)
{
account.Blocked = true;
}
}
protected List<Account> Accounts = new List<Account>
{
new Account {Id = 1, FirstName = "John", LastName = "Smith", ExpireDate = DateTime.Now.AddYears(1), Blocked = false},
new Account {Id = 2, FirstName = "Sarah", LastName = "Johnson", ExpireDate = DateTime.Now.AddYears(1), Blocked = false},
new Account {Id = 3, FirstName = "Michael", LastName = "Brown", ExpireDate = DateTime.Now.AddYears(1), Blocked = false},
new Account {Id = 4, FirstName = "Emily", LastName = "Davis", ExpireDate = DateTime.Now.AddYears(1), Blocked = false}
};
public class Account {
public int Id { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
public DateTime ExpireDate { get; set; }
public bool Blocked { get; set; }
}
}
We could use the custom Blazor data grid that we created in a previous post to display the data in a table.
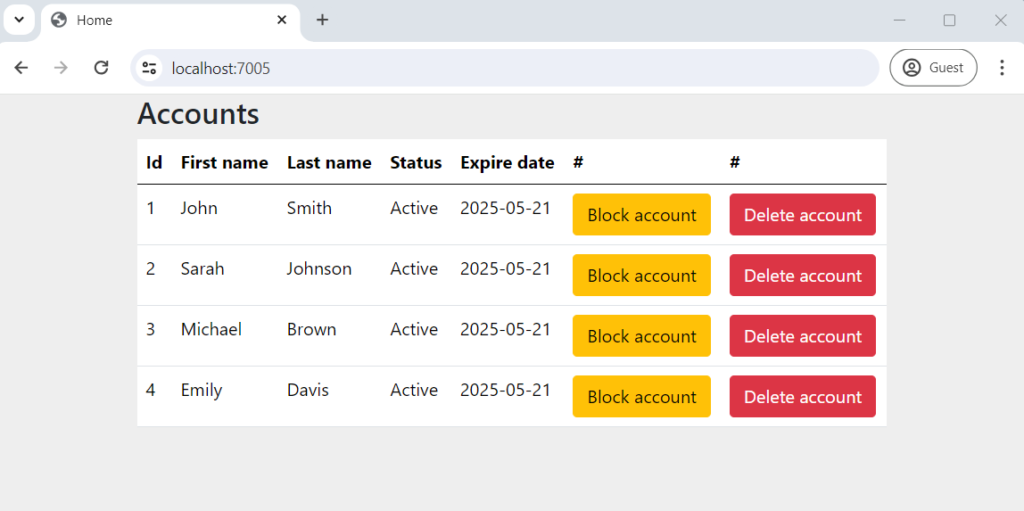
Try to delete or block an account and observe the Blazor confirm dialog in action.
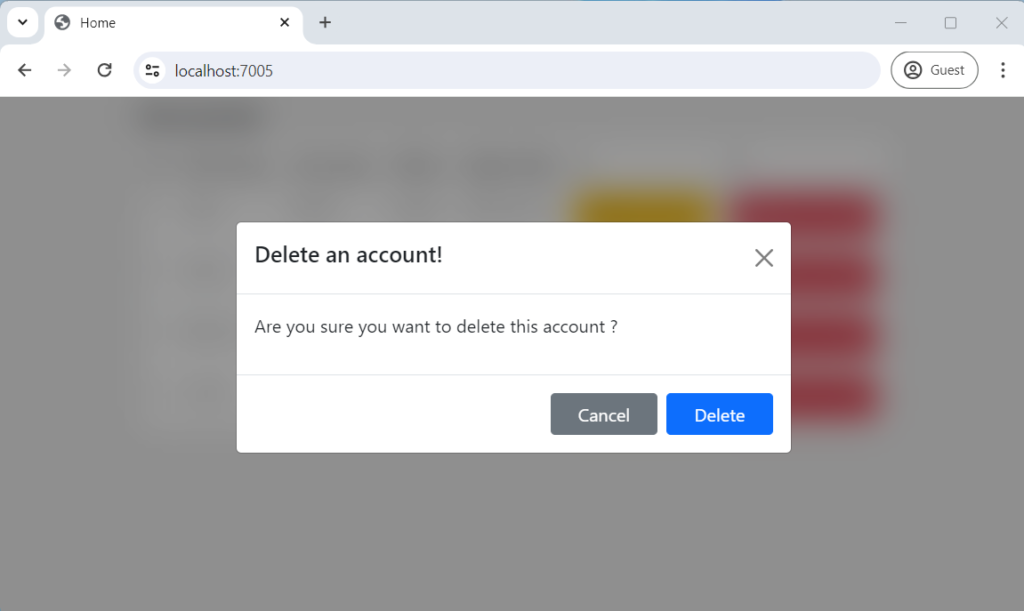
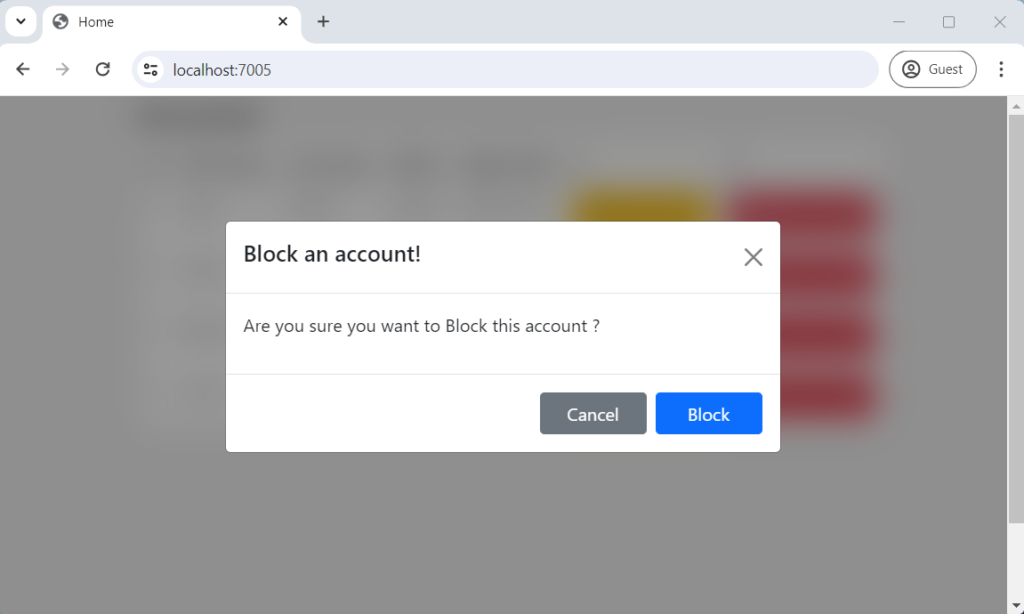
Conclusion
We created a Blazor Confirm dialog component in this post that you may utilize in your different projects. We started by creating a Blazor class library and then, we added the Blazor confirm dialog component code behind and the mark up and finally we had a look at the Blazor confirm dialog component in use.
The code for the Blazor Toast component can be found Here.
Also read https://dotnetcoder.com/creating-a-blazor-toast-component-using-c-only-html-and-css/
Enjoy This Blog?
Discover more from Dot Net Coder
Subscribe to get the latest posts sent to your email.